C++ Exercises: Count the number of strings with a given length in an array
Count Strings of Given Length in String Array
Write a C++ program to count the number of strings with a given length in a given array of strings.
Test Data:
({"a", "b", "bb", "c", "ccc" }, 1) ->3
({"a", "b", "bb", "c", "ccc" }, 2) ->1
({"a", "b", "bb", "c", "ccc" }, 3) ->1
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <string.h> // Including string manipulation header file
using namespace std; // Using standard namespace
// Function to count strings of a particular length in an array of strings
int test(string arr_str[], int len, int arr_length) {
int ctr = 0; // Counter variable to store the count of strings with the specified length
// Loop through the array of strings
for (int i = 0; i < arr_length; i++) {
if (arr_str[i].size() == len) // Check if the length of the string matches the specified length
ctr++; // Increment the counter if the length matches
}
return ctr; // Return the count of strings with the specified length
}
// Main function
int main() {
// Array of strings
string text[] = {
"a",
"b",
"bb",
"c",
"ccc"
};
// Displaying original array strings
cout << "Original array strings: ";
int arr_length = sizeof(text) / sizeof(text[0]);
for (size_t i = 0; i < arr_length; i++) {
std::cout << text[i] << ' ';
}
// Finding and displaying the count of strings with different lengths
int len = 1;
cout << "\n\nNumber of strings with length " << len << " = " << test(text, len, arr_length) << endl;
len = 2;
cout << "\nNumber of strings with length " << len << " = " << test(text, len, arr_length) << endl;
len = 3;
cout << "\nNumber of strings with length " << len << " = " << test(text, len, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
Original array strings: a b bb c ccc Number of strings with length 1 = 3 Number of strings with length 2 = 1 Number of strings with length 3 = 1
Flowchart:
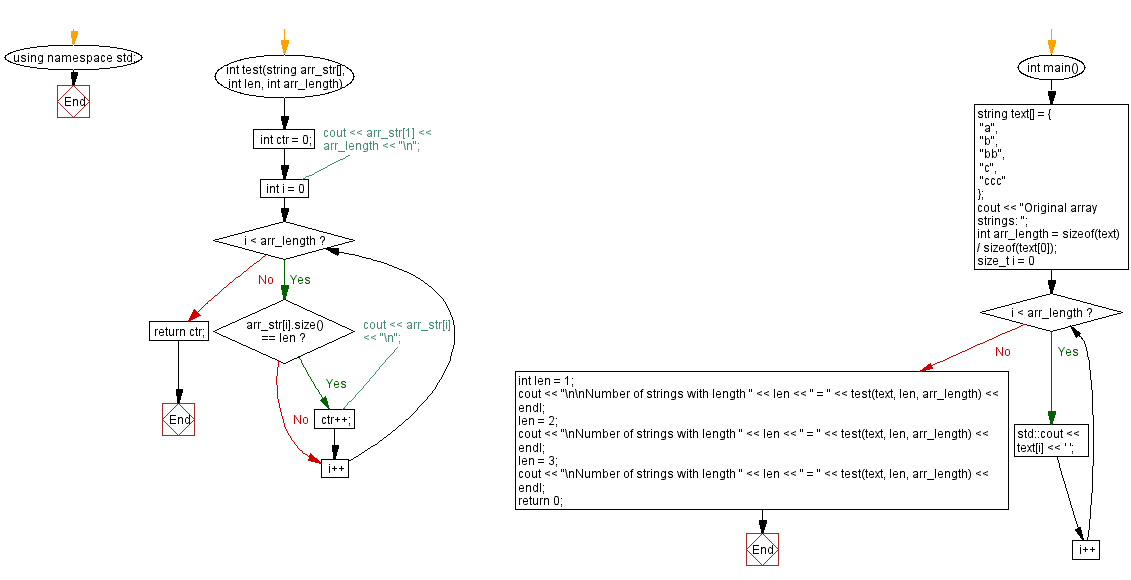
For more Practice: Solve these Related Problems:
- Write a C++ program to count the number of strings of a specified length within an array of strings and output the count.
- Write a C++ program that reads an array of strings and a target length, then prints how many strings have that exact length.
- Write a C++ program to iterate over a string array and count the elements whose length matches a given number.
- Write a C++ program that accepts an array of strings and a length value, then returns the total number of strings with that length.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Find the largest average value between the first and second halves of a given array of integers.
Next: New array from an existing array.
What is the difficulty level of this exercise?