C++ Exercises: Check if a given string starts with 'C#' or not
C++ Basic Algorithm: Exercise-12 with Solution
Check If String Starts with 'C#'
Write a C++ program to check if a given string starts with 'C#' or not.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check conditions based on the input string
bool test(string str)
{
// Check if the string satisfies one of the specified conditions
return (str.length() < 3 && str == "C#") || (str.substr(0, 2) == "C#" && str[2] == ' ');
}
// Main function
int main()
{
cout << test("C# Sharp") << endl; // Output the result of test function with string "C# Sharp"
cout << test("C#") << endl; // Output the result of test function with string "C#"
cout << test("C++") << endl; // Output the result of test function with string "C++"
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
1 1 0
Visual Presentation:
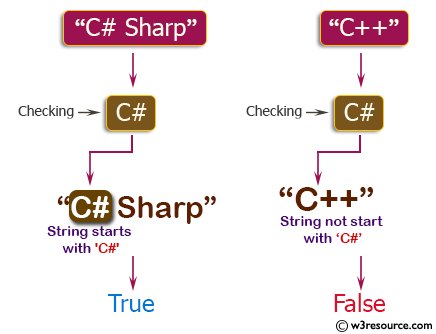
Flowchart:
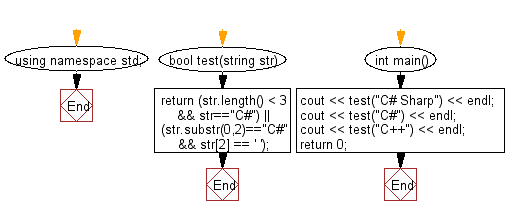
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string taking the first 3 characters of a given string and return the string with the 3 characters added at both the front and back. If the given string length is less than 3, use whatever characters are there.
Next: Write a C++ program to check if one given temperatures is less than 0 and the other is greater than 100.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-12.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics