C++ Exercises: Check if one given temperatures is less than 0 and the other is greater than 100
Temperature Less than 0 or Greater than 100
Write a C++ program to check if one given temperature is less than 0 and the other is greater than 100.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check temperature conditions
bool test(int temp1, int temp2)
{
// Check if the given temperature values satisfy specific conditions
return temp1 < 0 && temp2 > 100 || temp2 < 0 && temp1 > 100;
}
// Main function
int main()
{
cout << test(120, -1) << endl; // Output the result of test function with temperatures 120 and -1
cout << test(-1, 120) << endl; // Output the result of test function with temperatures -1 and 120
cout << test(2, 120) << endl; // Output the result of test function with temperatures 2 and 120
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
1 1 0
Visual Presentation:
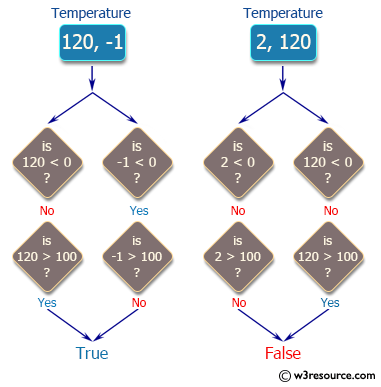
Flowchart:
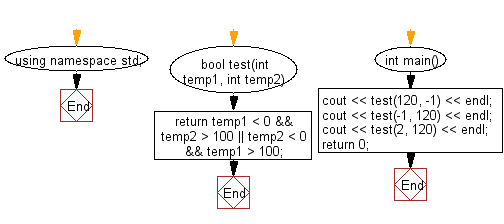
For more Practice: Solve these Related Problems:
- Write a C++ program that accepts two temperature values and returns true if one is below 0 and the other is above 100.
- Write a C++ program to check if one temperature is sub-zero while the other exceeds 100, printing the boolean result.
- Write a C++ program that reads two temperatures and outputs true only if one is less than 0 and the other greater than 100.
- Write a C++ program to validate a pair of temperatures, ensuring one is under freezing point and the other above boiling point.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if a given string starts with 'C#' or not.
Next: Write a C++ program to check two given integers whether either of them is in the range 100..200 inclusive.
What is the difficulty level of this exercise?