C++ Exercises: Test a positive integer and return true if it contains the number 2
C++ Basic Algorithm: Exercise-122 with Solution
Check if Number Contains Digit '3'
Write a C++ program to check a positive integer and return true if it contains the number 3. Otherwise return false.
Test Data:
(143) -> 1
(678) -> 0
(963) -> 1
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function to check if a given integer contains the digit 3
bool test(int n) {
while (n > 0) {
if (n % 10 == 3) // Checking if the last digit of 'n' is 3
return true; // Returning true if 'n' contains the digit 3
n /= 10; // Removing the last digit of 'n' by integer division
}
return false; // Returning false if 'n' does not contain the digit 3
}
// Main function
int main() {
int n = 143; // Initializing 'n' with an integer value
cout << "Original number: " << n; // Displaying the original number
cout << "\nCheck the said integer contains 3? " << test(n); // Checking if 'n' contains the digit 3
n = 678; // Changing the value of 'n' to a different integer
cout << "\n\nOriginal number: " << n; // Displaying the new original number
cout << "\nCheck the said integer contains 3? " << test(n); // Checking if 'n' contains the digit 3
n = 963; // Changing the value of 'n' to another integer
cout << "\n\nOriginal number: " << n; // Displaying the new original number
cout << "\nCheck the said integer contains 3? " << test(n); // Checking if 'n' contains the digit 3
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
Original number: 143 Check the said integer contains 3? 1 Original number: 678 Check the said integer contains 3? 0 Original number: 963 Check the said integer contains 3? 1
Flowchart:
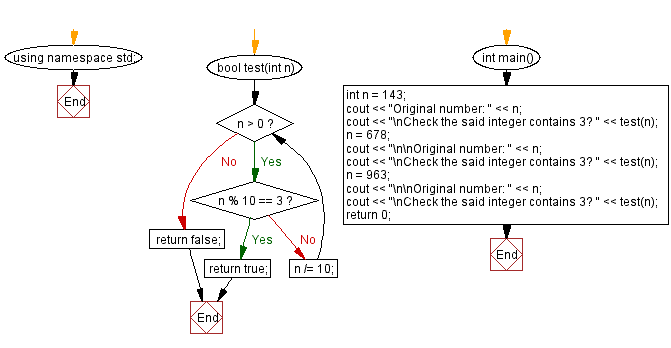
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Array from an existing array using string length.
Next: Create an array of odd numbers and specific lengths from an array.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-122.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics