C++ Exercises: Array from an existing array using string length
Create Array with Strings of Given Length from String Array
Write a C++ program to create an array from a given array of strings using all the strings whose lengths are matched with the given string length.
Test Data:
({a,aaa,b,bb,bbb,c,cc,ccc},1) -> {a,b,c}
({a,aaa,b,bb,bbb,c,cc,ccc},2) -> {bb, cc}
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include<string.h> // Including string manipulation header file
using namespace std; // Using standard namespace
string *new_array; // Declaring a pointer for the new string array
int ctr = 0; // Counter variable to track strings of a certain length
// Function to create a new string array containing strings of a given length
string *test(string *text, int arr_length, int n) {
int i = 0, j = 0; // Loop control variables
// Counting the number of strings with length 'n' in the input array 'text'
for (i = 0; i < arr_length; i++) {
if (text[i].size() == n)
ctr++; // Incrementing counter for strings of length 'n'
}
new_array = new string[ctr]; // Allocating memory for the new string array
// Copying strings of length 'n' from the input array 'text' to the new array 'new_array'
for (i = 0; i < arr_length; i++) {
if (text[i].size() == n) {
new_array[j] = text[i]; // Copying the string of length 'n' to 'new_array'
j++; // Incrementing index of the new array
}
}
return new_array; // Returning the pointer to the new string array
}
// Main function
int main() {
string text[] = {"a", "aaa", "b", "bb", "bbb", "c", "cc", "ccc"}; // Original array of strings
string *result_array; // Pointer to store the returned new string array
int arr_length = sizeof(text) / sizeof(text[0]); // Calculating the length of the original array
cout << "Original array elements: " << endl;
// Displaying the original array elements
for (int i = 0; i < arr_length; i++) {
std::cout << text[i] << ' ';
}
int n = 1; // String length to filter the new array
result_array = test(text, arr_length, n); // Calling 'test' function to create a new array
cout << "\n\nNew array with given string length = " << n << endl;
// Displaying the new array containing strings of length 'n'
for (int i = 0; i < ctr; i++) {
std::cout << result_array[i] << ' ';
}
n = 2; // Changing the string length to filter the new array
result_array = test(text, arr_length, n); // Creating a new array with updated string length
cout << "\n\nNew array with given string length = " << n << endl;
// Displaying the new array containing strings of length 'n'
for (int i = 0; i < ctr; i++) {
std::cout << result_array[i] << ' ';
}
delete[] new_array; // Deleting the allocated memory for the new array
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
Original array elements: a aaa b bb bbb c cc ccc New array with given string length = 1 a b c New array with given string length = 2 bb cc
Flowchart:
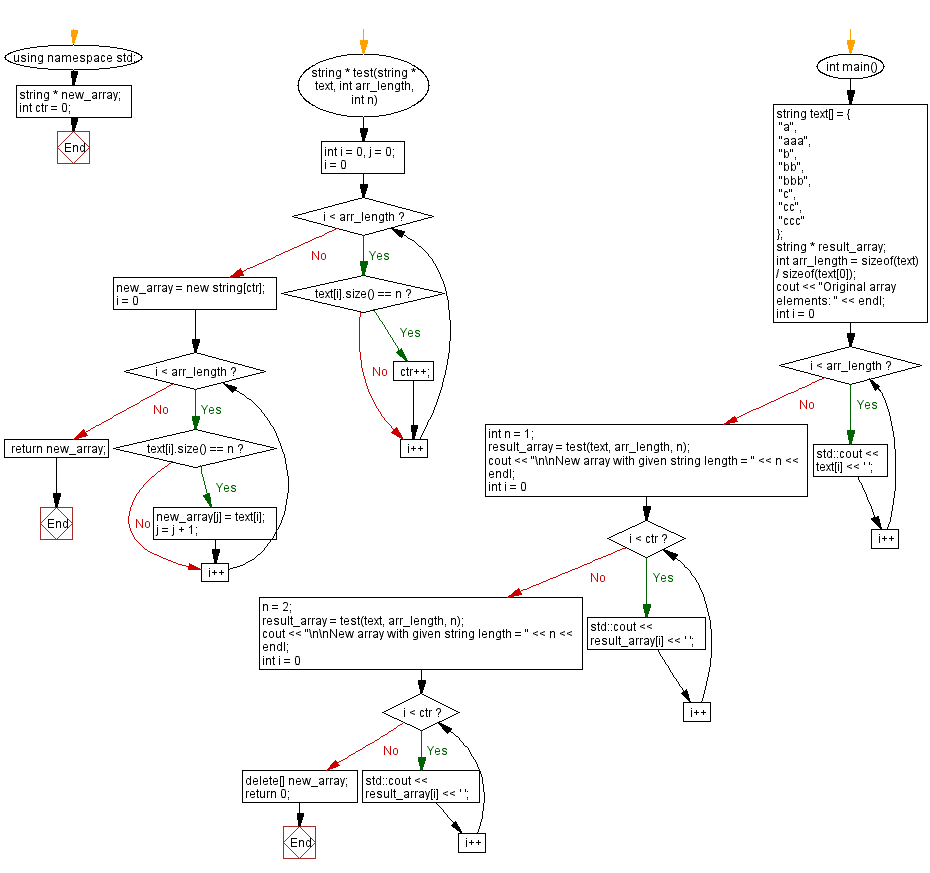
For more Practice: Solve these Related Problems:
- Write a C++ program to filter a string array and create a new array containing only strings that match a specified length.
- Write a C++ program that reads an array of strings and a target length, then outputs an array of strings having that exact length.
- Write a C++ program to process an array of strings and extract all elements whose lengths equal a given number.
- Write a C++ program that accepts a list of strings and a desired length, then forms a new array with only those strings meeting the length criteria.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: New array from an existing array.
Next: Test a positive integer and return true if it contains the number 2.
What is the difficulty level of this exercise?