C++ Exercises: Check whether three given integer values are in the range 20..50 inclusive
C++ Basic Algorithm: Exercise-15 with Solution
Check if Integers are in Range 20–50
Write a C++ program to check whether three given integer values are in the range 20..50 inclusive. Return true if 1 or more of them are in the range, otherwise false.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if any of the given integers falls within the range 20 to 50 (inclusive)
bool test(int x, int y, int z)
{
// Check if any of x, y, or z is within the range 20 to 50
return (x >= 20 && x <= 50) || (y >= 20 && y <= 50) || (z >= 20 && z <= 50);
}
// Main function
int main()
{
cout << test(11, 20, 12) << endl; // Output the result of test function with values 11, 20, and 12
cout << test(30, 30, 17) << endl; // Output the result of test function with values 30, 30, and 17
cout << test(25, 35, 50) << endl; // Output the result of test function with values 25, 35, and 50
cout << test(15, 12, 8) << endl; // Output the result of test function with values 15, 12, and 8
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
1 1 1 0
Visual Presentation:
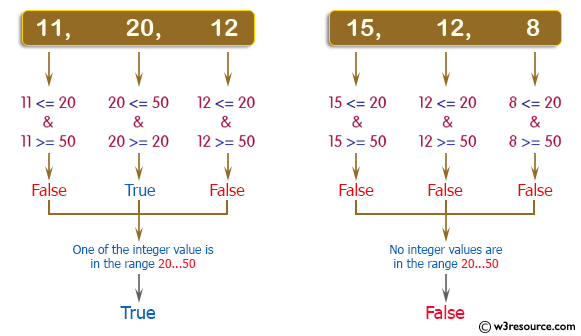
Flowchart:
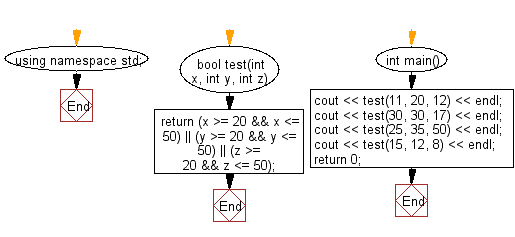
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check two given integers whether either of them is in the range 100..200 inclusive.
Next: Write a C++ program to check whether two given integer values are in the range 20..50 inclusive. Return true if 1 or other is in the said range otherwise false.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-15.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics