C++ Exercises: Check whether two given integer values are in the range 20..50 inclusive
Check if Either Integer is in Range 20–50
Write a C++ program to check whether two given integer values are in the range 20..50 inclusive. Return true if 1 or other is in the range, otherwise false.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check conditions for two integer values
bool test(int x, int y)
{
// Check if x is less than or equal to 20 or y is greater than or equal to 50,
// or if y is less than or equal to 20 or x is greater than or equal to 50
return (x <= 20 || y >= 50) || (y <= 20 || x >= 50);
}
// Main function
int main()
{
cout << test(20, 84) << endl; // Output the result of test function with values 20 and 84
cout << test(14, 50) << endl; // Output the result of test function with values 14 and 50
cout << test(11, 45) << endl; // Output the result of test function with values 11 and 45
cout << test(25, 40) << endl; // Output the result of test function with values 25 and 40
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
1 1 1 0
Visual Presentation:
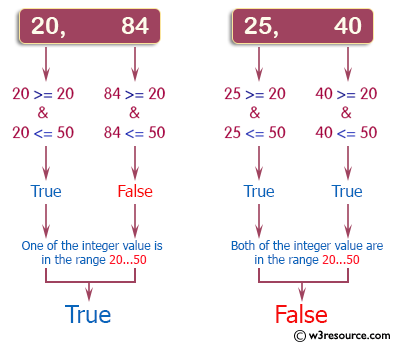
Flowchart:
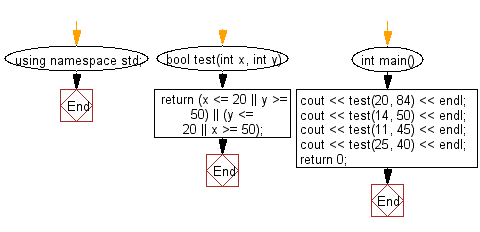
For more Practice: Solve these Related Problems:
- Write a C++ program to check two integers and return true if at least one of them is between 20 and 50 inclusive.
- Write a C++ program that reads two numbers and outputs true if either is within the range 20–50, false otherwise.
- Write a C++ program to determine if one of two provided integers lies in the inclusive range of 20 to 50 and prints the result.
- Write a C++ program that evaluates two integer inputs and returns a boolean value indicating if one of them is within 20–50.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check whether three given integer values are in the range 20..50 inclusive. Return true if 1 or more of them are in the said range otherwise false.
Next: Write a C++ program to check if a string 'yt' appears at index 1 in a given string. If it appears return a string without 'yt' otherwise return the original string.
What is the difficulty level of this exercise?