C++ Exercises: Check the largest number among three given integers
C++ Basic Algorithm: Exercise-18 with Solution
Write a C++ program to check the largest number among three given integers.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to find the maximum value among three integers
int test(int x, int y, int z)
{
// Calculate the maximum value among x, y, and z using the max function from the standard library
int result = max(x, max(y, z));
return result; // Return the maximum value
}
// Main function
int main()
{
cout << test(1,2,3) << endl; // Output the result of test function with values 1, 2, and 3
cout << test(1,3,2) << endl; // Output the result of test function with values 1, 3, and 2
cout << test(1,1,1) << endl; // Output the result of test function with values 1, 1, and 1
cout << test(1,2,2) << endl; // Output the result of test function with values 1, 2, and 2
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
3 3 1 2
Visual Presentation:
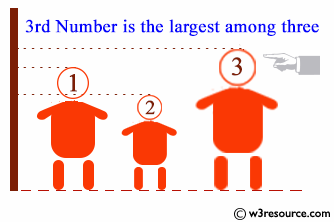
Flowchart:
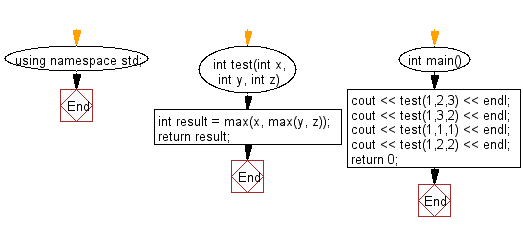
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if a string 'yt' appears at index 1 in a given string. If it appears return a string without 'yt' otherwise return the original string.
Next: Write a C++ program to check which number nearest to the value 100 among two given integers. Return 0 if the two numbers are equal.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics