C++ Exercises: Check which number nearest to the value 100 among two given integers
Closest to 100
Write a C++ program to check which number is closest to the value 100 among two given integers. Return 0 if the two numbers are equal.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to determine the number closer to 100 among two integers
int test(int x, int y)
{
const int n = 100; // Constant integer n is set to 100
// Calculate the absolute difference between x and n, and between y and n
int val = abs(x - n);
int val2 = abs(y - n);
// Check if the absolute differences are equal or not
// If equal, return 0; otherwise, return the number closest to 100
return val == val2 ? 0 : (val < val2 ? x : y);
}
// Main function
int main()
{
cout << test(78, 95) << endl; // Output the result of test function with values 78 and 95
cout << test(95, 95) << endl; // Output the result of test function with values 95 and 95
cout << test(99, 70) << endl; // Output the result of test function with values 99 and 70
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
95 0 99
Visual Presentation:
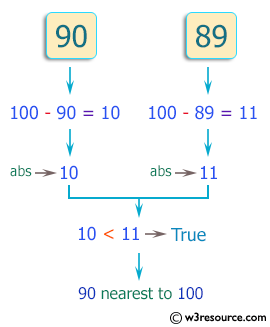

Flowchart:
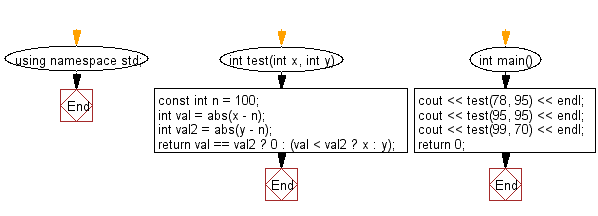
For more Practice: Solve these Related Problems:
- Write a C++ program to compare two integers and return the one that is closest to 100, or 0 if they are equal.
- Write a C++ program that reads two numbers and outputs the one nearer to 100, handling edge cases where the difference is the same.
- Write a C++ program to determine which of two input values is closest to 100, using absolute difference calculations.
- Write a C++ program that compares two numbers to see which is nearer to 100, and prints 0 if both are equidistant.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check the largest number among three given integers.
Next: Write a C++ program to check whether two given integers are in the range 40..50 inclusive, or they are both in the range 50..60 inclusive.
What is the difficulty level of this exercise?