C++ Exercises: Count the number of two 5's are next to each other in an array of integers
Count Consecutive 5's in Array
Write a C++ program to count the number of 5's next to each other in an array of integers. Count the situation where the second 5 is actually a 6.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to count occurrences where the array contains either (5, 5) or (5, 6) pairs
int test(int numbers[], int arr_length)
{
int ctr = 0;
// Loop through the array
for (int i = 0; i < arr_length; i++)
{
// Check for pairs (5, 5) or (5, 6)
if ((numbers[i] == 5 && numbers[i + 1] == 5) || (numbers[i] == 5 && numbers[i + 1] == 6))
{
ctr++; // Increment counter when a valid pair is found
}
}
return ctr; // Return the count of valid pairs
}
int main()
{
int arr_length;
// Test cases with different integer arrays
int nums1[] = {5, 5, 2};
arr_length = sizeof(nums1) / sizeof(nums1[0]);
cout << test(nums1, arr_length) << endl;
int nums2[] = {5, 5, 2, 5, 5};
arr_length = sizeof(nums2) / sizeof(nums2[0]);
cout << test(nums2, arr_length) << endl;
int nums3[] = {5, 6, 2, 9};
arr_length = sizeof(nums3) / sizeof(nums3[0]);
cout << test(nums3, arr_length) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
1 2 1
Visual Presentation:
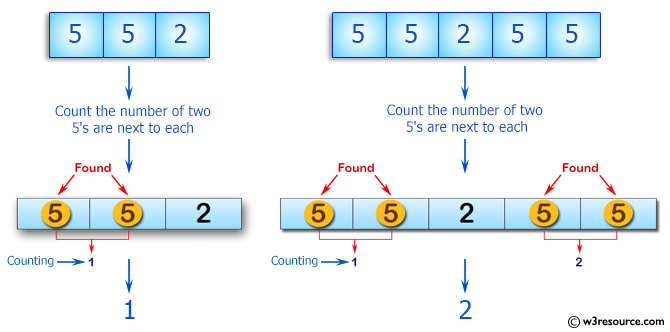
Flowchart:
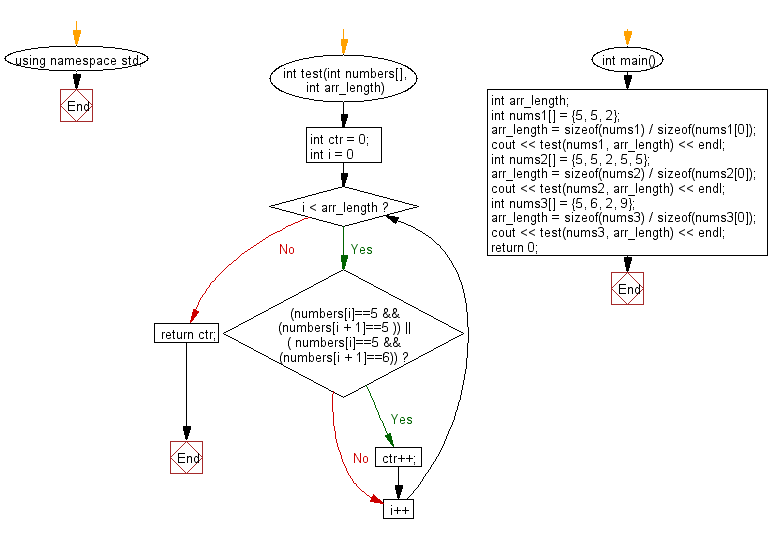
For more Practice: Solve these Related Problems:
- Write a C++ program to count the number of occurrences where two 5's appear consecutively in an array, treating a 6 following a 5 as a valid pair.
- Write a C++ program that scans an integer array and counts adjacent pairs of 5’s, with the special condition that a 6 can substitute the second 5.
- Write a C++ program to iterate through an array and output the count of consecutive 5's, considering a 5 followed by a 6 as a match.
- Write a C++ program that reads an array of integers and returns the number of times a 5 is immediately followed by either another 5 or a 6.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string from a give string where a specified character have been removed except starting and ending position of the given string.
Next: Write a C++ program to check if a triple is presents in an array of integers or not. If a value appears three times in a row in an array it is called a triple.
What is the difficulty level of this exercise?