C++ Exercises: Check if a triple is presents in an array of integers or not
Check for Triple in Array
Write a C++ program to check if a triple is present in an array of integers or not. If a value appears three times in a row in an array it is called a triple.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if there are three consecutive identical numbers in the array
bool test(int nums[], int arr_length)
{
int arra_len = arr_length - 1; // Length of the array - 1
int n = 0;
for (int i = 0; i < arra_len; i++)
{
n = nums[i]; // Current element in the iteration
// Check if the current element and the next two elements are identical
if (n == nums[i + 1] && n == nums[i + 2])
{
return true; // Return true if three consecutive identical numbers are found
}
}
return false; // Return false if no such sequence is found in the array
}
int main()
{
int arr_length;
// Test cases with different integer arrays
int nums1[] = {1, 1, 2, 2, 1};
arr_length = sizeof(nums1) / sizeof(nums1[0]);
cout << test(nums1, arr_length) << endl;
int nums2[] = {1, 1, 2, 1, 2, 3};
arr_length = sizeof(nums2) / sizeof(nums2[0]);
cout << test(nums2, arr_length) << endl;
int nums3[] = {1, 1, 1, 2, 2, 2, 1};
arr_length = sizeof(nums3) / sizeof(nums3[0]);
cout << test(nums3, arr_length) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
0 0 1
Visual Presentation:
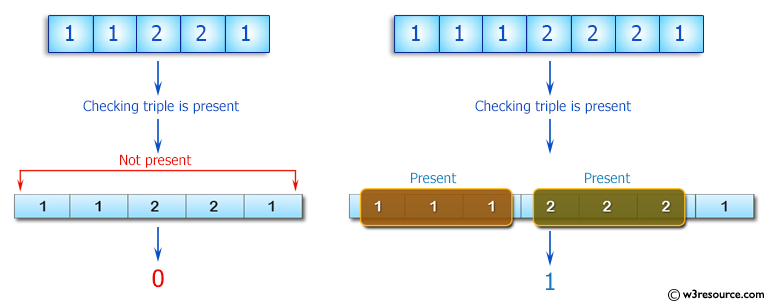
Flowchart:
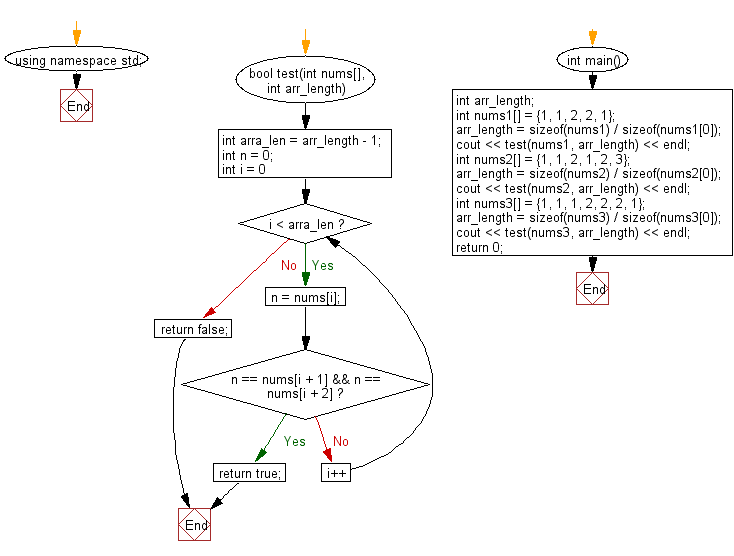
For more Practice: Solve these Related Problems:
- Write a C++ program to check if any integer appears three times consecutively in an array and return true if found.
- Write a C++ program that reads an array and outputs a boolean value indicating whether a triple (three same numbers in a row) exists.
- Write a C++ program to detect if any element in an array is repeated three times in succession, using loop-based comparisons.
- Write a C++ program that processes an array of integers and prints true if it encounters a sequence where the same value occurs three times consecutively.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to count the number of two 5's are next to each other in an array of integers. Also count the situation where the second 5 is actually a 6.
Next: Write a C++ program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
What is the difficulty level of this exercise?