C++ Exercises: Compute the sum of the two given integers, if the sum is in the range 10..20 inclusive return 30
Sum of Integers, Return 30 in Range 10–20
Write a C++ program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to calculate and return a modified sum based on certain conditions
int test(int a, int b)
{
// Check if the sum of 'a' and 'b' is between 10 and 20 inclusive
// If true, return 30; otherwise, return the sum of 'a' and 'b'
return (a + b >= 10 && a + b <= 20) ? 30 : a + b;
}
int main()
{
// Test cases to check different scenarios of the test function
cout << test(12, 17) << endl;
cout << test(2, 17) << endl;
cout << test(22, 17) << endl;
cout << test(20, 0) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
29 30 39 30
Visual Presentation:
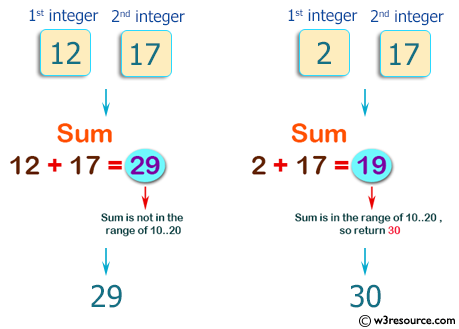
Flowchart:
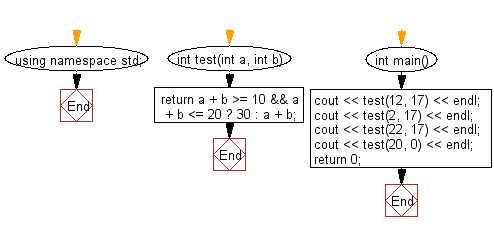
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the sum of two integers, but if the sum falls within the range 10 to 20 inclusive, output 30 instead.
- Write a C++ program that reads two numbers, calculates their sum, and returns 30 if the sum is between 10 and 20, otherwise returns the sum.
- Write a C++ program to add two integers and check if the result lies between 10 and 20, in which case it outputs 30, else prints the computed sum.
- Write a C++ program that conditionally modifies the sum of two numbers: if the sum is in the range 10–20, return 30; otherwise, return the original sum.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if a triple is presents in an array of integers or not. If a value appears three times in a row in an array it is called a triple.
Next: Write a C++ program that accept two integers and return true if either one is 5 or their sum or difference is 5.
What is the difficulty level of this exercise?