C++ Exercises: Test if a given non-negative number is a multiple of 13 or it is one more than a multiple of 13
C++ Basic Algorithm: Exercise-39 with Solution
Write a C++ program to test if a given non-negative number is a multiple of 13 or it is one more than a multiple of 13.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if a number is either divisible by 13 or has a remainder of 1 when divided by 13
bool test(int n)
{
// Returns true if the number n is divisible by 13 or has a remainder of 1 when divided by 13
return n % 13 == 0 || n % 13 == 1;
}
int main()
{
// Test cases to check different scenarios of the test function
cout << test(13) << endl; // Output: 1 (true)
cout << test(14) << endl; // Output: 1 (true)
cout << test(27) << endl; // Output: 1 (true)
cout << test(41) << endl; // Output: 0 (false)
return 0; // Return 0 to indicate successful completion
}
Sample Output:
1 1 1 0
Flowchart:
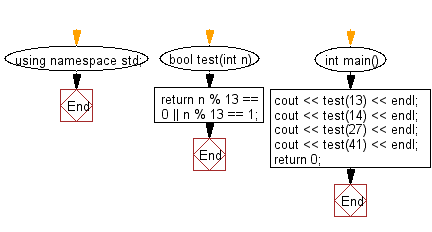
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program that accept two integers and return true if either one is 5 or their sum or difference is 5.
Next: Write a C++ program to check if a given non-negative number is a multiple of 3 or 7, but not both.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics