C++ Exercises: Check if a given non-negative number is a multiple of 3 or 7, but not both
Multiple of 3 or 7 but Not Both
Write a C++ program to check if a given number that is not negative is a multiple of 3 or 7, but not both.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to determine if a number is divisible by 3 or 7 exclusively (but not both)
bool test(int n)
{
// Returns true if n is divisible by 3 exclusively XOR (n is divisible by 7 exclusively)
return n % 3 == 0 ^ n % 7 == 0;
}
int main()
{
// Testing the test function with different input values
cout << test(3) << endl; // Output: 1 (true), divisible by 3 exclusively
cout << test(7) << endl; // Output: 1 (true), divisible by 7 exclusively
cout << test(21) << endl; // Output: 0 (false), divisible by both 3 and 7
return 0; // Return 0 to indicate successful completion
}
Sample Output:
1 1 0
Visual Presentation:
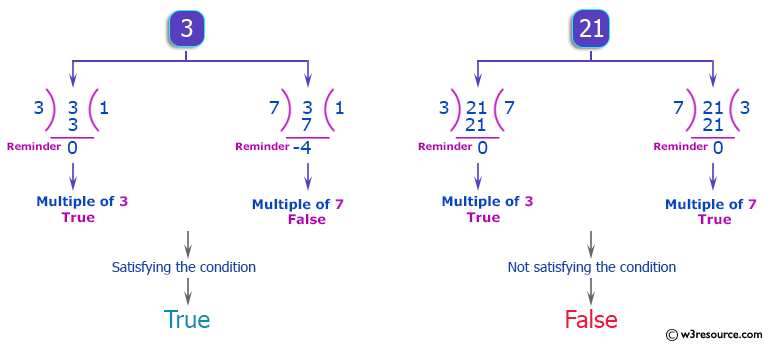
Flowchart:
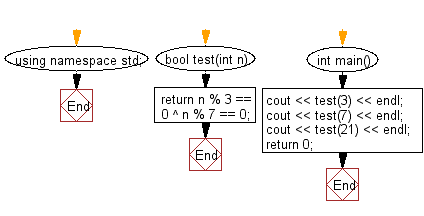
For more Practice: Solve these Related Problems:
- Write a C++ program to check if a non-negative number is a multiple of 3 or 7, but not a multiple of both, and print true or false.
- Write a C++ program that reads a number and verifies it is divisible by either 3 or 7 exclusively, using logical operators to avoid dual multiples.
- Write a C++ program to determine if an input number is a multiple of 3 or 7 while ensuring it is not divisible by both simultaneously.
- Write a C++ program that checks a number’s divisibility by 3 and 7 separately and outputs true only if exactly one of these conditions holds.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to test if a given non-negative number is a multiple of 13 or it is one more than a multiple of 13.
Next: Write a C++ program to check if a given number is within 2 of a multiple of 10.
What is the difficulty level of this exercise?