C++ Exercises: Check if it is possible to add two integers to get the third integer from three given integers
C++ Basic Algorithm: Exercise-44 with Solution
Add Two Integers to Get Third
Write a C++ program to check if it is possible to add two integers to get the third integer from three given integers.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if the sum of any two numbers equals the third number
bool test(int x, int y, int z)
{
return x == y + z || y == x + z || z == x + y;
}
int main()
{
// Testing the 'test' function with different sets of numbers
cout << test(1, 2, 3) << endl;
cout << test(4, 5, 6) << endl;
cout << test(-1, 1, 0) << endl;
return 0;
}
Sample Output:
1 0 1
Visual Presentation:
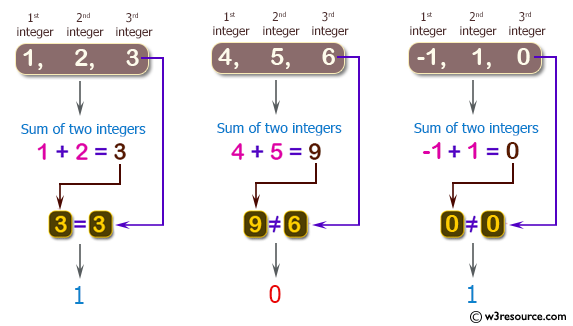
Flowchart:
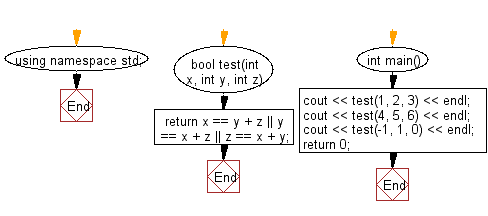
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check whether a given string starts with "F" or ends with "B". If the string starts with "F" return "Fizz" and return "Buzz" if it ends with "B" If the string starts with "F" and ends with "B" return "FizzBuzz". In other cases return the original string.
Next: Write a C++ program to check if y is greater than x, and z is greater than y from three given integers x,y,z.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-44.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics