C++ Exercises: Check whether a given string starts with "F" or ends with "B"
Check String Starts with 'F' or Ends with 'B'
Write a C++ program to check whether a given string starts with "F" or ends with "B". If the string starts with "F" return "Fizz" and return "Buzz" if it ends with "B" If the string starts with "F" and ends with "B" return "FizzBuzz". In other cases return the original string.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to process a string according to specific conditions
string test(string str)
{
// Check if the string starts with 'F' and ends with 'B'
if ((str.substr(0, 1) == "F") && (str.substr(str.length() - 1, 1) == "B"))
{
return "FizzBuzz"; // Return "FizzBuzz" if conditions are met
}
// Check if the string starts with 'F'
else if (str.substr(0, 1) == "F")
{
return "Fizz"; // Return "Fizz" if the string starts with 'F'
}
// Check if the string ends with 'B'
else if (str.substr(str.length() - 1, 1) == "B")
{
return "Buzz"; // Return "Buzz" if the string ends with 'B'
}
else
{
return str; // Return the original string if none of the conditions are met
}
}
int main()
{
// Test cases for the test function
cout << test("FB") << endl; // Output: FizzBuzz (starts with 'F' and ends with 'B')
cout << test("Fsafs") << endl; // Output: Fizz (starts with 'F')
cout << test("AuzzB") << endl; // Output: Buzz (ends with 'B')
cout << test("founder") << endl;// Output: founder (no condition met)
return 0;
}
Sample Output:
FizzBuzz Fizz Buzz founder
Visual Presentation:
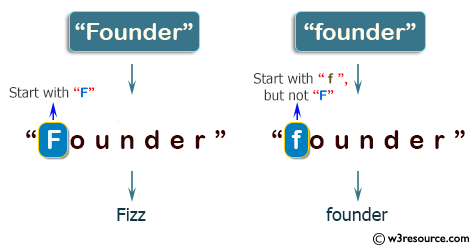
Flowchart:
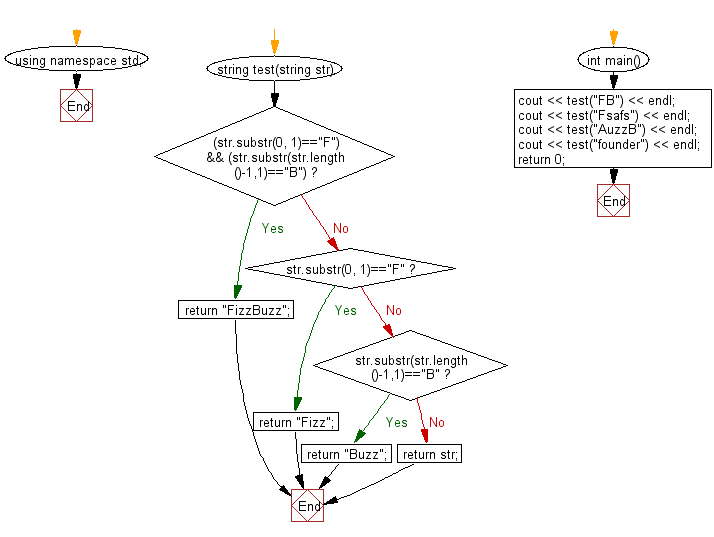
For more Practice: Solve these Related Problems:
- Write a C++ program to analyze a string and return "Fizz" if it starts with 'F', "Buzz" if it ends with 'B', and "FizzBuzz" if both conditions are met; otherwise, return the original string.
- Write a C++ program that checks an input string for a starting 'F' and an ending 'B' and prints a modified string accordingly.
- Write a C++ program to process a string and output "Fizz", "Buzz", or "FizzBuzz" based on its first and last characters, or the unchanged string if none match.
- Write a C++ program that reads a string and applies conditional logic to prepend "Fizz" or append "Buzz" depending on whether it starts with 'F' or ends with 'B'.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to compute the sum of the two given integers. If one of the given integer value is in the range 10..20 inclusive return 18.
Next: Write a C++ program to check if it is possible to add two integers to get the third integer from three given integers.
What is the difficulty level of this exercise?