C++ Exercises: Find the larger from two given integers
Larger Integer or Smaller with Same Remainder Modulo 7
Write a C++ program to find the larger of two given integers. However if the two integers have the same remainder when divided by 7, then return the smaller integer. If the two integers are the same, return 0.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' takes two integers (x, y) as parameters
int test(int x, int y)
{
// Checks if x is equal to y
if (x == y)
{
return 0; // Returns 0 if x is equal to y
}
// Checks conditions for returning x or y based on specific cases
else if ((x % 7 == y % 7 && x < y) || x > y)
{
return x; // Returns x if x%7 equals y%7 and x is less than y, or if x is greater than y
}
else
{
return y; // Returns y in all other cases
}
}
int main()
{
// Testing the 'test' function with different sets of numbers
cout << test(11, 21) << endl;
cout << test(11, 20) << endl;
cout << test(10, 10) << endl;
return 0;
}
Sample Output:
21 20 0
Visual Presentation:
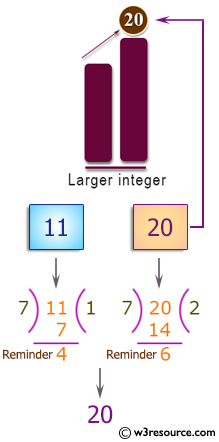
Flowchart:
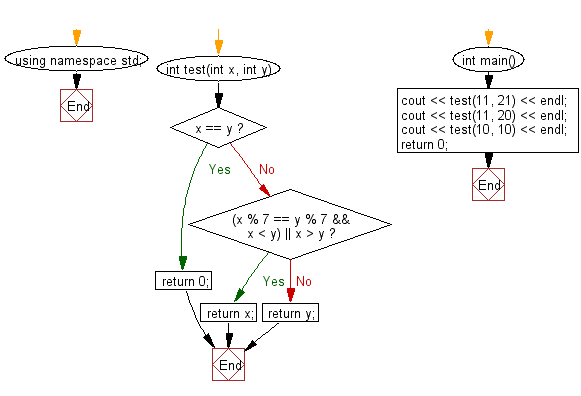
For more Practice: Solve these Related Problems:
- Write a C++ program to compare two integers and return the larger one unless they have the same remainder when divided by 7, in which case return the smaller.
- Write a C++ program that reads two numbers and checks their modulo 7 values to decide whether to return the larger or smaller integer.
- Write a C++ program to determine the larger of two integers, but if they yield the same remainder upon division by 7, output the lower value.
- Write a C++ program that compares two integers and, based on their remainders modulo 7, either prints the larger or, if equal modulo 7, prints the smaller; if they are equal, print 0.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check three given integers and return true if one of them is 20 or more less than one of the others.
Next: Write a C++ program to check two given integers, each in the range 10..99. Return true if a digit appears in both numbers, such as the 3 in 13 and 33.
What is the difficulty level of this exercise?