C++ Exercises: Create a new string where 'if' is added to the front of a given string
C++ Basic Algorithm: Exercise-5 with Solution
Add 'if' to String
Write a C++ program to create a string where 'if' is added to the front of a given string. If the string already begins with 'if', return the string unchanged.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to modify a string based on certain conditions
string test(string s)
{
// Check if the string length is greater than 2 and if the substring from index 0 to 1 (first two characters) is "if"
if (s.length() > 2 && s.substr(0, 2) == "if")
{
return s; // Return the string as it is if it starts with "if" and has a length greater than 2
}
return "if " + s; // Prepend "if " to the string if it doesn't start with "if" or has a length less than or equal to 2
}
// Main function
int main()
{
cout << test("if else") << endl; // Output the result of test function with argument "if else"
cout << test("else") << endl; // Output the result of test function with argument "else"
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
if else if else
Visual Presentation:
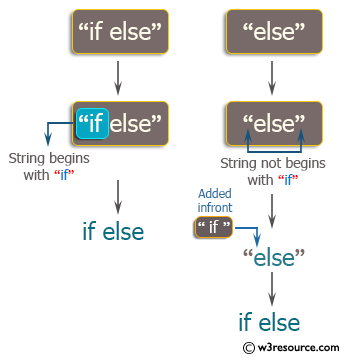
Flowchart:
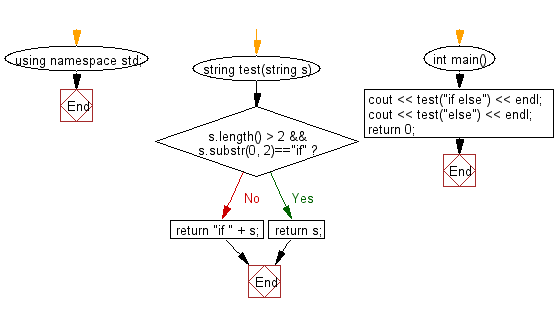
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given integer and return true if it is within 10 of 100 or 200.
Next: Write a C++ program to remove the character in a given position of a given string. The given position will be in the range 0..string length -1 inclusive.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics