C++ Exercises: Remove the character in a given position of a given string
Remove Character from String
Write a C++ program to remove the character at a given position in the string. The given position will be in the range 0..string length -1 inclusive.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to remove a character at index 'n' from the string 'str'
string test(string str, int n)
{
return str.erase(n, 1); // Erase one character at index 'n' from the string 'str' and return the modified string
}
// Main function
int main()
{
cout << test("Python", 1) << endl; // Output the result of test function with string "Python" and index 1
cout << test("Python", 0) << endl; // Output the result of test function with string "Python" and index 0
cout << test("Python", 4) << endl; // Output the result of test function with string "Python" and index 4
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
Pthon ython Pythn
Pictorial Presentation:
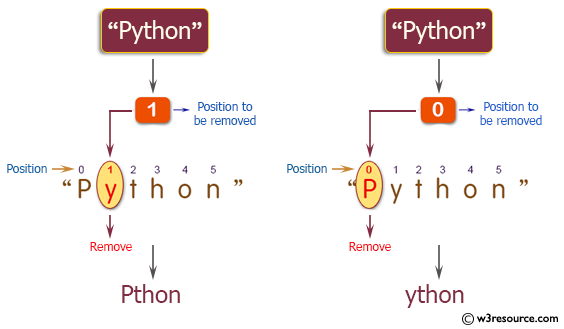
Flowchart:
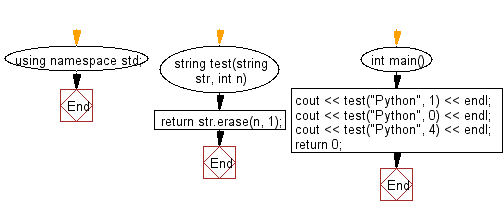
For more Practice: Solve these Related Problems:
- Write a C++ program to remove a character from a string at a specified index provided by the user.
- Write a C++ program that takes a string and an index, and then outputs the string after deleting the character at that position.
- Write a C++ program to delete the character at a given position in a string, ensuring proper bounds checking for the index.
- Write a C++ program that accepts a string and a position, then removes the character at that index and prints the new string.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string where 'if' is added to the front of a given string. If the string already begins with 'if', return the string unchanged.
Next: Write a C++ program to exchange the first and last characters in a given string and return the new string.
What is the difficulty level of this exercise?