C++ Exercises: Exchange the first and last characters in a given string and return the new string
Swap First and Last Characters
Write a C++ program to exchange the first and last characters in a given string and return the result string.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to modify a string based on certain conditions
string test(string str)
{
// Check if the length of the string is greater than 1
return str.length() > 1
? str.substr(str.length() - 1) + str.substr(1, str.length() - 2) + str.substr(0, 1) // Rearrange the string by moving the first character to the end
: str; // Return the string as it is if its length is 1 or less
}
// Main function
int main()
{
cout << test("abcd") << endl; // Output the result of test function with string "abcd"
cout << test("a") << endl; // Output the result of test function with string "a"
cout << test("xy") << endl; // Output the result of test function with string "xy"
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
dbca a yx
Visual Presentation:
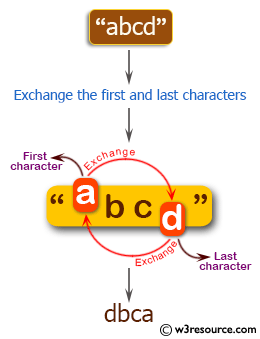
Flowchart:
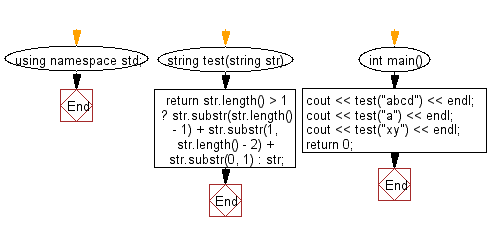
For more Practice: Solve these Related Problems:
- Write a C++ program that exchanges the first and last characters of a string and outputs the resulting string.
- Write a C++ program to swap the first and last letters of an input string, handling edge cases for strings of length one.
- Write a C++ program that reads a string and interchanges its first and last characters before printing the modified string.
- Write a C++ program that takes a string input and, using pointer manipulation, swaps its first and last characters.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to remove the character in a given position of a given string. The given position will be in the range 0..string length -1 inclusive.
Next: Write a C++ program to create a new string which is 4 copies of the 2 front characters of a given string. If the given string length is less than 2 return the original string.
What is the difficulty level of this exercise?