C++ Exercises: Create a new string which is 4 copies of the 2 front characters of a given string
C++ Basic Algorithm: Exercise-8 with Solution
4 Copies of First 2 Characters
Write a C++ program to create a string that is 4 copies of the 2 front characters of a given string. If the given string length is less than 2 return the original string.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to modify a string based on certain conditions
string test(string str)
{
// Check if the length of the string is less than 2
return str.length() < 2 ? str : // If the length is less than 2, return the string as it is
str.substr(0, 2) + str.substr(0, 2) + str.substr(0, 2) + str.substr(0, 2); // If the length is 2 or more, concatenate the first two characters of the string four times
}
// Main function
int main()
{
cout << test("C Sharp") << endl; // Output the result of test function with string "C Sharp"
cout << test("JS") << endl; // Output the result of test function with string "JS"
cout << test("a") << endl; // Output the result of test function with string "a"
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
C C C C JSJSJSJS a
Visual Presentation:
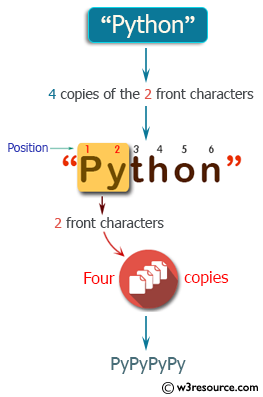
Flowchart:
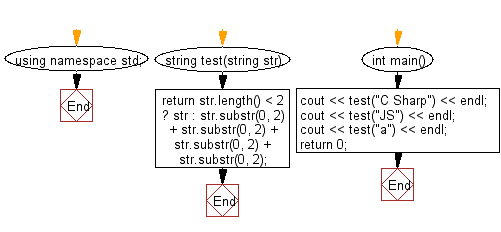
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to exchange the first and last characters in a given string and return the new string.
Next: Write a C++ program to create a new string with the last char added at the front and back of a given string of length 1 or more.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics