C++ Exercises: Create a new string using first two characters of a given string
First Two Characters or Full String if Less Than 2
Write a C++ program to create a new string using the first two characters of a given string. If the string length is less than 2, return the original string.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' returns the first two characters of the input string if its length is 2 or more
// Otherwise, it returns the entire input string
string test(string s1)
{
// Checks if the length of the input string is less than 2
if (s1.length() < 2)
{
// Returns the input string 's1' if its length is less than 2
return s1;
}
else
{
// Returns the first two characters of the input string 's1'
return s1.substr(0, 2);
}
}
// Main function to test the 'test' function
int main()
{
// Displays the output of the 'test' function for different string inputs
cout << test("Hello") << endl; // Output: "He" (first two characters of "Hello")
cout << test("Hi") << endl; // Output: "Hi" (entire string as length is 2)
cout << test("H") << endl; // Output: "H" (entire string as length is less than 2)
cout << test(" ") << endl; // Output: " " (entire string as length is less than 2)
return 0;
}
Sample Output:
He Hi H
Visual Presentation:
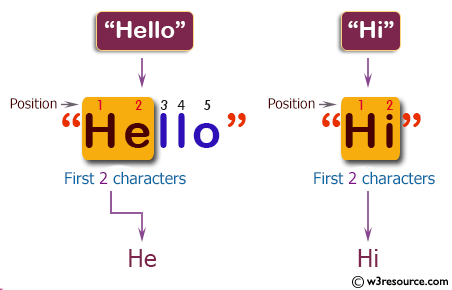
Flowchart:
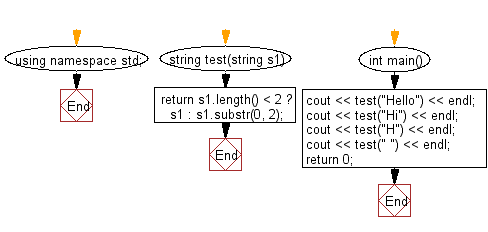
For more Practice: Solve these Related Problems:
- Write a C++ program to output the first two characters of a string, or the entire string if its length is less than two.
- Write a C++ program that reads a string and prints its first two letters; if the string is too short, print it as is.
- Write a C++ program to extract and display the first two characters of an input string, with a condition for short strings.
- Write a C++ program that checks the length of a string and, if it is under two characters, outputs the full string; otherwise, outputs the first two characters.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string using three copies of the last two character of a given string of length atleast two.
Next: Write a C++ program to create a new string of the first half of a given string of even length.
What is the difficulty level of this exercise?