C++ Exercises: Create a new string of the first half of a given string of even length
First Half of Even-Length String
Write a C++ program to create the string of the first half of a given string of even length.
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Use the standard namespace
// Function definition to return the first half of the input string
string test(string s1) // Function named 'test' accepting a string parameter 's1'
{
return s1.substr(0, s1.length() / 2); // Return a substring from index 0 to half the length of the input string 's1'
}
// Main function - entry point of the program
int main()
{
cout << test("Hello") << endl;
cout << test("Hi") << endl;
return 0; // Indicate successful completion of the program
}
Sample Output:
He H
Visual Presentation:
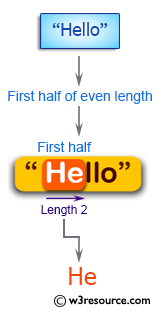
Flowchart:
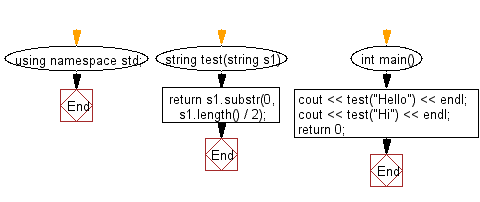
For more Practice: Solve these Related Problems:
- Write a C++ program to obtain and print the first half of a string with an even number of characters.
- Write a C++ program that reads an even-length string and outputs the substring representing its first half.
- Write a C++ program to split a string of even length into two equal parts and print the first part.
- Write a C++ program that takes an even-length string as input and extracts the first half using substring functions.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string using first two characters of a given string. If the string length is less than 2 then return the original string.
Next:Write a C++ program to create a new string without the first and last character of a given string of length atleast two.
What is the difficulty level of this exercise?