C++ Exercises: Create a new string from two given string one is shorter and another is longer
C++ Basic Algorithm: Exercise-63 with Solution
Long+Short+Long String Combination
Write a C++ program to create a new string from two given strings, one of which is shorter and the other is larger. The format of the updated string will be long string + short string + long string.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that concatenates strings based on their lengths
string test(string s1, string s2)
{
// Conditional operator to determine which string concatenation to perform
return s1.length() < s2.length() ? s2 + s1 + s2 : s1 + s2 + s1;
}
// Main function
int main()
{
cout << test("Hello", "Hi") << endl; // Output the result of test function with "Hello" and "Hi"
cout << test("JS", "Python") << endl; // Output the result of test function with "JS" and "Python"
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
HelloHiHello PythonJSPython
Visual Presentation:
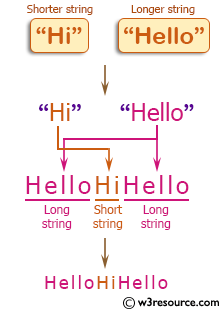
Flowchart:
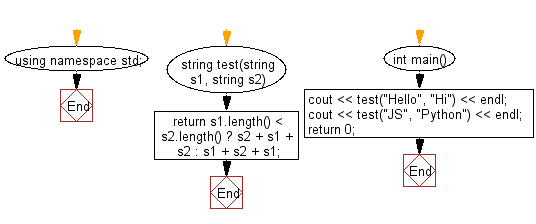
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string without the first and last character of a given string of length atleast two.
Next: Write a C++ program to concat two given string of length atleast 1, after removing their first character.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-63.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics