C++ Exercises: Concat two given string of length atleast 1, after removing their first character
Combine Strings Without First Characters
Write a C++ program to combine two strings of length at least 1, after removing their first character.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that concatenates substrings excluding the first character of each input string
string test(string s1, string s2)
{
return s1.substr(1) + s2.substr(1); // Concatenating s1 and s2 substrings starting from index 1
}
// Main function
int main()
{
cout << test("Hello", "Hi") << endl; // Output the result of test function with "Hello" and "Hi"
cout << test("JS", "Python") << endl; // Output the result of test function with "JS" and "Python"
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
elloi Sython
Visual Presentation:
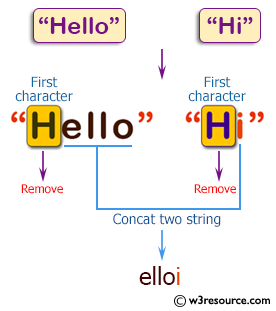
Flowchart:
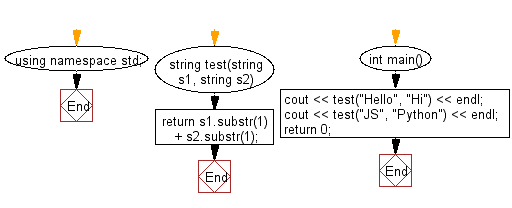
For more Practice: Solve these Related Problems:
- Write a C++ program to combine two strings after removing their first characters and output the resulting concatenation.
- Write a C++ program that reads two strings and prints a new string formed by joining them without their initial characters.
- Write a C++ program to strip the first letter from two input strings and concatenate the remaining parts into a single output string.
- Write a C++ program that takes two strings and outputs their combination after deleting the first character of each.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string from two given string one is shorter and another is longer. The format of the new string will be long string + short string + long string.
Next: Write a C++ program to move the first two characters to the end of a given string of length at least two.
What is the difficulty level of this exercise?