C++ Exercises: Move the first two characters to the end of a given string of length at least two
Move First Two Characters to End
Write a C++ program to move the first two characters to the end of a given string of length at least two.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that manipulates input string and returns a modified string
string test(string s1)
{
string s2 = s1; // Declaring and assigning s2 as a copy of s1
return s1.erase(0, 2) + s2.substr(0, 2); // Erase the first two characters of s1 and concatenate them with the first two characters of s2
}
// Main function
int main()
{
cout << test("Hello") << endl; // Output the result of test function with "Hello"
cout << test("JS") << endl; // Output the result of test function with "JS"
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
lloHe JS
Visual Presentation:
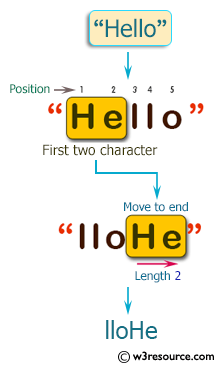
Flowchart:
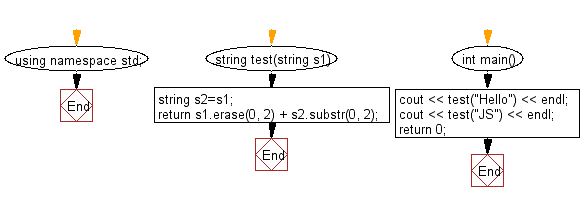
For more Practice: Solve these Related Problems:
- Write a C++ program to shift the first two characters of a string to its end and output the resulting string.
- Write a C++ program that reads a string and moves its initial two characters to the end, handling strings of exact length two appropriately.
- Write a C++ program to process a string by removing its first two characters and appending them at the end before printing the new string.
- Write a C++ program that accepts a string input and rearranges it by taking the first two letters and placing them at the string's end.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to concat two given string of length atleast 1, after removing their first character.
Next: Write a C++ program to create a new string without the first and last character of a given string of any length.
What is the difficulty level of this exercise?