C++ Exercises: Create a new string from a given string after swapping last two characters
Swap Last Two Characters
Write a C++ program to create a new string from a given string after swapping the last two characters.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that performs manipulation on the input string
string test(string s1)
{
// Check if the length of the string is greater than 1
if (s1.length() > 1)
{
// Return a modified string formed by rearranging characters:
// Substring from index 0 to length-2 (excluding last two characters)
// Last character of the original string
// Second-to-last character of the original string
return s1.substr(0, s1.length() - 2) + s1[s1.length() - 1] + s1[s1.length() - 2];
}
else
{
// Return the original string if its length is 1 or less
return s1;
}
}
// Main function
int main()
{
// Output the results of test function with different input strings
cout << test("Hello") << endl; // Output for "Hello"
cout << test("Python") << endl; // Output for "Python"
cout << test("PHP") << endl; // Output for "PHP"
cout << test("JS") << endl; // Output for "JS"
cout << test("C") << endl; // Output for "C"
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
Helol Pythno PPH SJ C
Visual Presentation:
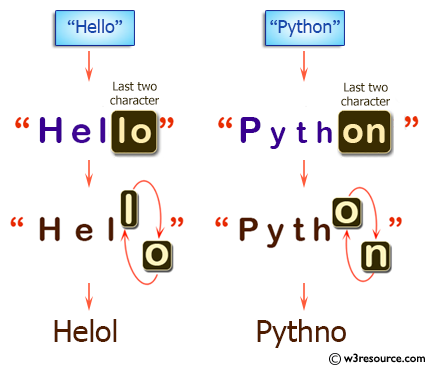
Flowchart:
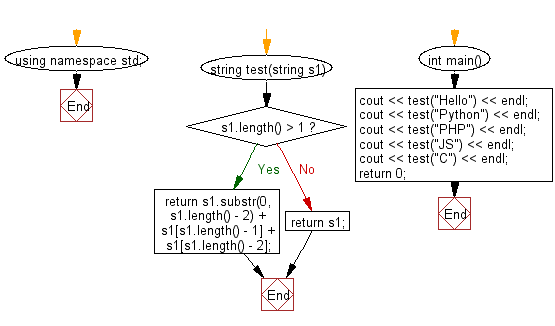
For more Practice: Solve these Related Problems:
- Write a C++ program that reads a string and swaps its last two characters, handling cases where the string length is less than 2 by returning the original string.
- Write a C++ program to exchange the positions of the final two characters of an input string, ensuring proper handling of short strings.
- Write a C++ program that takes a string input and outputs a new string with the last two characters interchanged; if the string length is one, print it as is.
- Write a C++ program to reverse the order of the final two letters of a string and print the modified string, leaving strings of insufficient length unchanged.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string taking the first character from a given string and the last character from another given string. If the length of any given string is 0, use '#' as its missing character.
Next: Write a C++ program to check if a given string begins with 'abc' or 'xyz'. If the string begins with 'abc' or 'xyz' return 'abc' or 'xyz' otherwise return the empty string.
What is the difficulty level of this exercise?