C++ Exercises: Check if a given string begins with 'abc' or 'xyz'
Starts with 'abc' or 'xyz', Return It or Empty
Write a C++ program to check if a given string begins with 'abc' or 'xyz'. If the string begins with 'abc' or 'xyz' return 'abc' or 'xyz' otherwise return the empty string.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that checks the starting substring of the input string
string test(string s1)
{
// Check if the first three characters of s1 are "abc"
if (s1.substr(0, 3) == "abc")
{
return "abc"; // Return "abc" if the first three characters match "abc"
}
// Check if the first three characters of s1 are "xyz"
else if (s1.substr(0, 3) == "xyz")
{
return "xyz"; // Return "xyz" if the first three characters match "xyz"
}
else
{
return ""; // Return an empty string if neither "abc" nor "xyz" is found at the beginning
}
}
// Main function
int main()
{
// Output the results of test function with different input strings
cout << test("abc") << endl; // Output for "abc"
cout << test("abcdef") << endl; // Output for "abcdef"
cout << test("C") << endl; // Output for "C"
cout << test("xyz") << endl; // Output for "xyz"
cout << test("xyzsder") << endl; // Output for "xyzsder"
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
abc abc xyz xyz
Visual Presentation:
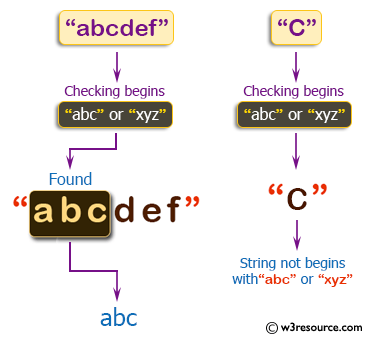
Flowchart:
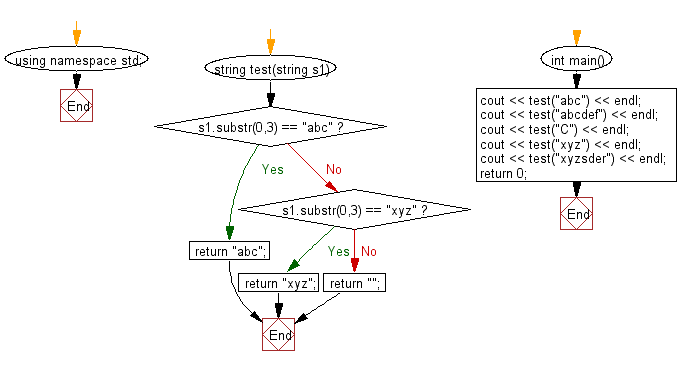
For more Practice: Solve these Related Problems:
- Write a C++ program that checks whether an input string begins with "abc" or "xyz" and returns that substring; if not, return an empty string.
- Write a C++ program to verify the prefix of a string for "abc" or "xyz" and output the matching prefix or nothing if no match is found.
- Write a C++ program that reads a string and prints "abc" if it starts with "abc", "xyz" if it starts with "xyz", otherwise prints an empty string.
- Write a C++ program to examine an input string's beginning and return the substring "abc" or "xyz" when detected, returning a blank string if neither is present.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string from a given string after swapping last two characters.
Next: Write a C++ program to check whether the first two characters and last two characters of a given string are same.
What is the difficulty level of this exercise?