C++ Exercises: Check whether the first two characters and last two characters of a given string are same
First Two = Last Two Characters Check
Write a C++ program to check whether the first two characters and the last two characters of a given string are the same.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that checks if the first two characters are equal to the last two characters of the input string
bool test(string s1)
{
return s1.substr(0, 2) == s1.substr(s1.length() - 2); // Return true if the first two characters are equal to the last two characters of s1
}
// Main function
int main()
{
cout << test("abab") << endl; // Output for "abab"
cout << test("abcdef") << endl; // Output for "abcdef"
cout << test("xyzsderxy") << endl; // Output for "xyzsderxy"
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
1 0 1
Visual Presentation:
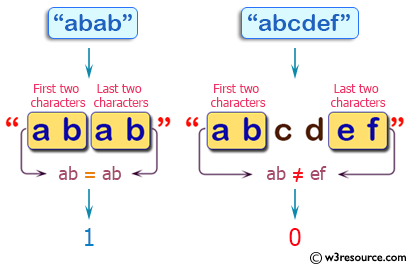
Flowchart:
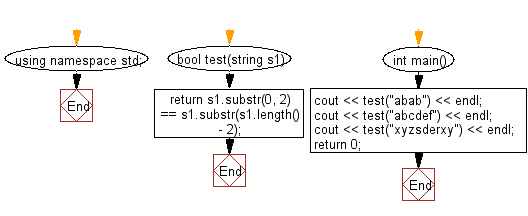
For more Practice: Solve these Related Problems:
- Write a C++ program to check if the first two characters of a string match its last two characters and output a boolean value accordingly.
- Write a C++ program that reads a string and returns true if the starting two letters are identical to the ending two letters, otherwise false.
- Write a C++ program to compare the first and last two characters of an input string and print 1 for a match or 0 otherwise.
- Write a C++ program that examines a string for equal leading and trailing pairs and outputs the result as a boolean value.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if a given string begins with 'abc' or 'xyz'. If the string begins with 'abc' or 'xyz' return 'abc' or 'xyz' otherwise return the empty string.
Next: Write a C++ program to concat two given strings. If the given strings have different length remove the characters from the longer string.
What is the difficulty level of this exercise?