C++ Exercises: Create a new string from a given string
Remove First Two Characters if Start = End
Write a C++ program to create a new string from a string. Return the given string without the first two characters if the two characters at the beginning and end are the same. Otherwise, return the original string.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that checks the first and last two characters of the input string and modifies it accordingly
string test(string s1)
{
// Check if the length of s1 is greater than 1 and the first two characters are equal to the last two characters
if (s1.length() > 1 && s1.substr(0, 2) == s1.substr(s1.length() - 2))
{
return s1.substr(2); // Return a substring of s1 starting from index 2
}
else
{
return s1; // Return s1 unchanged if the condition is not met
}
}
// Main function
int main()
{
cout << test("abcab") << endl; // Output for "abcab"
cout << test("Python") << endl; // Output for "Python"
cout << test("abcabab") << endl; // Output for "abcabab"
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
cab Python cabab
Visual Presentation:
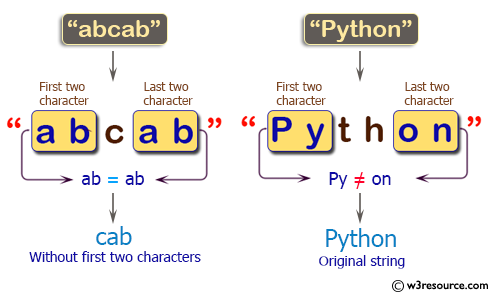
Flowchart:
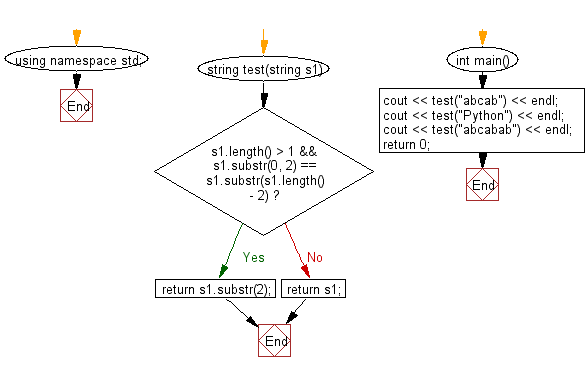
For more Practice: Solve these Related Problems:
- Write a C++ program that removes the first two characters from a string if they are identical to the last two characters; otherwise, return the original string.
- Write a C++ program to check whether the beginning and ending of a string match; if so, remove the first two characters and print the modified string.
- Write a C++ program that reads a string and deletes its first two characters if these match its final two characters, otherwise outputs the unchanged string.
- Write a C++ program to compare the first two and last two characters of a string, removing the leading pair if they are the same.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string using 3 copies of the first 2 characters of a given string. If the length of the given string is less than 2 use the whole string.
Next: Write a C++ program to create a new string from a given string without the first and last character if the first or last characters are 'a' otherwise return the original given string.
What is the difficulty level of this exercise?