C++ Exercises: Create a new string from a given string without the first and last character
Remove First/Last 'a' or Return Original
Write a C++ program to create a string from a given string without the first and last character. This is possible if the first or last characters are 'a' otherwise return the original given string.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that modifies the input string by removing 'a' from the beginning or end
string test(string s1)
{
// Check if the length of s1 is greater than 0 and the last character is 'a'
if (s1.length() > 0 && s1.substr(s1.length() - 1) == "a")
{
s1 = s1.erase(s1.length() - 1); // Remove the last character from s1
}
// Check if the length of s1 is greater than 0 and the first character is 'a'
if (s1.length() > 0 && s1.substr(0, 1) == "a")
{
s1 = s1.erase(0, 1); // Remove the first character from s1
}
return s1; // Return the modified string
}
// Main function
int main()
{
cout << test("abcab") << endl; // Output for "abcab"
cout << test("python") << endl; // Output for "python"
cout << test("abcda") << endl; // Output for "abcd"
cout << test("jython") << endl; // Output for "jython"
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
bcab python bcd jython
Visual Presentation:
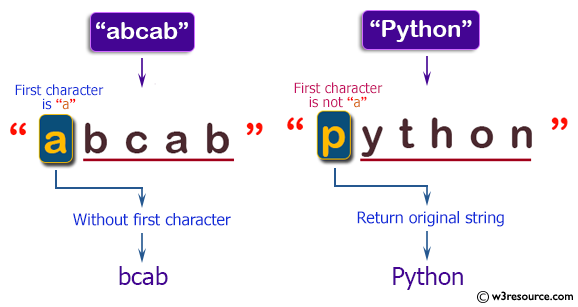
Flowchart:
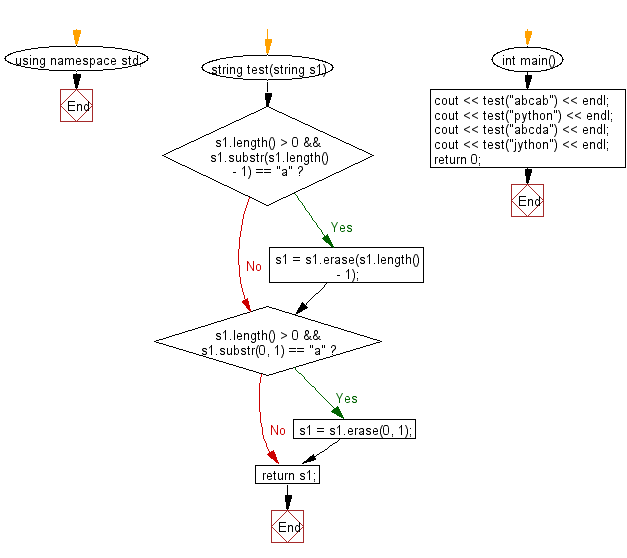
For more Practice: Solve these Related Problems:
- Write a C++ program to remove the first and/or last character from a string if they are 'a'; otherwise, return the string unchanged.
- Write a C++ program that reads a string and trims the character 'a' from the beginning and/or end if present, printing the result.
- Write a C++ program to check the first and last characters of a string for 'a' and remove them if found, else return the original string.
- Write a C++ program that conditionally strips 'a' from the boundaries of a string and outputs the modified string only when applicable.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string from a given string. If the two characters of the given string from its beginning and end are same return the given string without the first two characters otherwise return the original string.
Next: Write a C++ program to create a new string from a given string. If the first or first two characters is 'a', return the string without those 'a' characters otherwise return the original given string.
What is the difficulty level of this exercise?