C++ Exercises: Check a given array of integers of length 1 or more and return true if the first element and the last element are equal in the given array
First and Last Elements Match Check
Write a C++ program to check a given array of integers of length 1 or more. The program will return true if the first element and the last element are equal in the given array.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that checks if the first and last elements of an integer array are equal
bool test(int nums[], int arr_length)
{
return arr_length > 0 && nums[0] == nums[arr_length - 1];
// Return true if the array length is greater than 0 and the first element equals the last element
}
// Main function
int main()
{
int arr_length; // Declare variable to store array length
int nums1[] = {10, 20, 40, 50 }; // Define an array nums1
arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculate the length of nums1
cout << test(nums1, arr_length) << endl; // Output result of test function for nums1
int nums2[] = {10, 20, 40, 10}; // Define an array nums2
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculate the length of nums2
cout << test(nums2, arr_length) << endl; // Output result of test function for nums2
int nums4[] = {12, 24, 35, 55}; // Define an array nums4
arr_length = sizeof(nums4) / sizeof(nums4[0]); // Calculate the length of nums4
cout << test(nums4, arr_length) << endl; // Output result of test function for nums4
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
0 1 0
Visual Presentation:
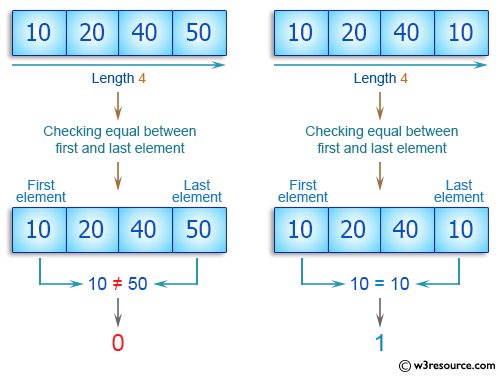
Flowchart:
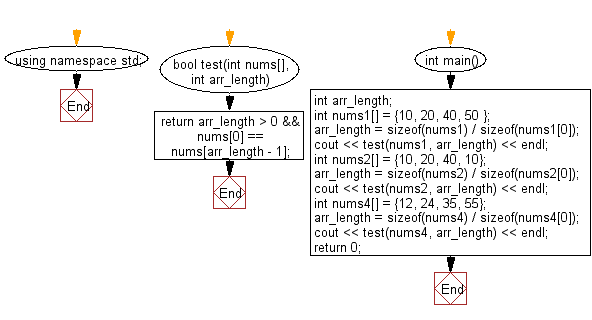
For more Practice: Solve these Related Problems:
- Write a C++ program to check if the first and last elements of an integer array are identical, returning a boolean value.
- Write a C++ program that reads an array and compares its first and last values, printing 1 if they match, otherwise 0.
- Write a C++ program to determine if the beginning and ending numbers of an array are the same and output the result.
- Write a C++ program that checks whether an array’s first and last elements are equal, using conditional statements to output true or false.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array of integers of length 1 or more and return true if 10 appears as either first or last element in the given array.
Next: Write a C++ program to check two given arrays of integers of length 1 or more and return true if they have the same first element or they have the same last element.
What is the difficulty level of this exercise?