C++ Exercises: Check two given arrays of integers of length 1 or more and return true if they have the same first element or they have the same last element
Same First or Last Element in Two Arrays
Write a C++ program to check two given arrays of integers of length 1 or more. This will return true if they have the same first element or if they have the same last element.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that checks if the first element of the first array matches the first element of the second array
// or if the last element of the first array matches the last element of the second array
bool test(int a1[], int arr_length1, int a2[], int arr_length2)
{
return a1[0] == a2[0] || a1[arr_length1 - 1] == a2[arr_length2 - 1];
// Return true if the first element of a1 matches the first element of a2
// or if the last element of a1 matches the last element of a2
}
// Main function
int main()
{
int arr_length1, arr_length2; // Declare variables to store array lengths
int nums11[] = {10, 20, 40, 50}; // Define an array nums11
int nums12[] = {10, 20, 40, 50}; // Define an array nums12
arr_length1 = sizeof(nums11) / sizeof(nums11[0]); // Calculate the length of nums11
arr_length2 = sizeof(nums12) / sizeof(nums12[0]); // Calculate the length of nums12
cout << test(nums11, arr_length1, nums12, arr_length2) << endl; // Output result of test function for nums11 and nums12
int nums21[] = {10, 20, 40, 50}; // Define an array nums21
int nums22[] = {10, 20, 40, 5}; // Define an array nums22
arr_length1 = sizeof(nums21) / sizeof(nums21[0]); // Calculate the length of nums21
arr_length2 = sizeof(nums22) / sizeof(nums22[0]); // Calculate the length of nums22
cout << test(nums11, arr_length1, nums12, arr_length2) << endl; // Output result of test function for nums21 and nums22
int nums31[] = {10, 20, 40, 50}; // Define an array nums31
int nums32[] = {1, 20, 40, 5}; // Define an array nums32
arr_length1 = sizeof(nums31) / sizeof(nums21[0]); // Calculate the length of nums31
arr_length2 = sizeof(nums32) / sizeof(nums22[0]); // Calculate the length of nums32
cout << test(nums31, arr_length1, nums32, arr_length2) << endl; // Output result of test function for nums31 and nums32
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
1 1 0
Visual Presentation:
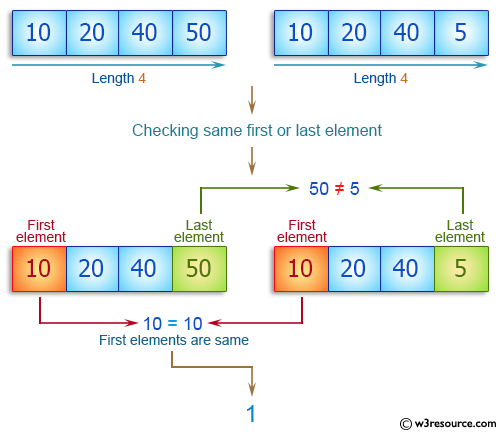
Flowchart:
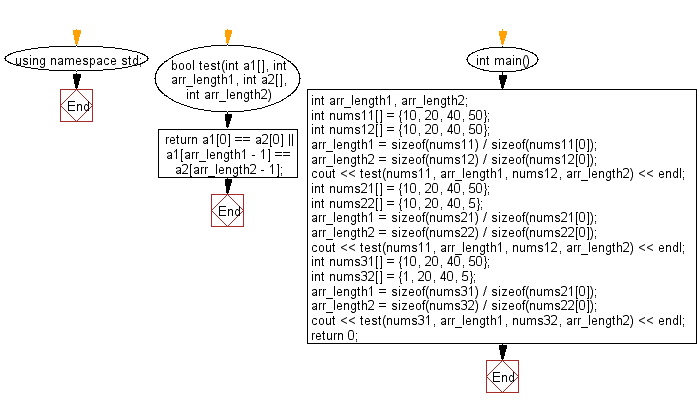
For more Practice: Solve these Related Problems:
- Write a C++ program to compare two arrays and return true if either their first elements match or their last elements match.
- Write a C++ program that reads two arrays and outputs true if the first element of one equals the first element of the other or if the last elements are equal.
- Write a C++ program to check if two integer arrays share a common first or last element, and print the result as a boolean.
- Write a C++ program that takes two arrays as input and verifies if their boundary elements (first or last) are the same, returning true if so.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array of integers of length 1 or more and return true if the first element and the last element are equal in the given array.
Next: Write a C++ program to compute the sum of the elements of a given array of integers.
What is the difficulty level of this exercise?