C++ Exercises: Rotate the elements of a given array of integers in left direction and return the new array
Left Rotate Array (Length 4)
Write a C++ program to rotate the elements of a given array of integers (length 4 ) in the left direction and return the changed array.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that rotates the elements of an array by one position to the left
int *test(int nums[]) {
// Static array storing the elements after rotation
static int r_array[] = { nums[1], nums[2], nums[3], nums[0] };
return r_array; // Returning the rotated array
}
// Main function
int main () {
int *p; // Pointer to an integer
int nums[] = {10, 20, 30, 40}; // Define an array nums
int arr_length = sizeof(nums) / sizeof(nums[0]); // Calculate the length of nums
cout << "Original array: " << endl;
for ( int i = 0; i < arr_length; i++ ) {
cout << nums[i] << " "; // Output the original array
}
p = test(nums); // Rotate the array and assign it to pointer p
cout << "\nRotated array: " << endl;
for ( int i = 0; i < arr_length; i++ ) {
cout << *(p + i) << " "; // Output the rotated array
}
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
Original array: 10 20 30 40 Rotated array: 20 30 40 10
Visual Presentation:
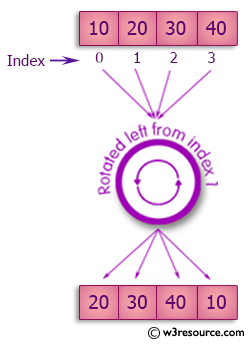
Flowchart:
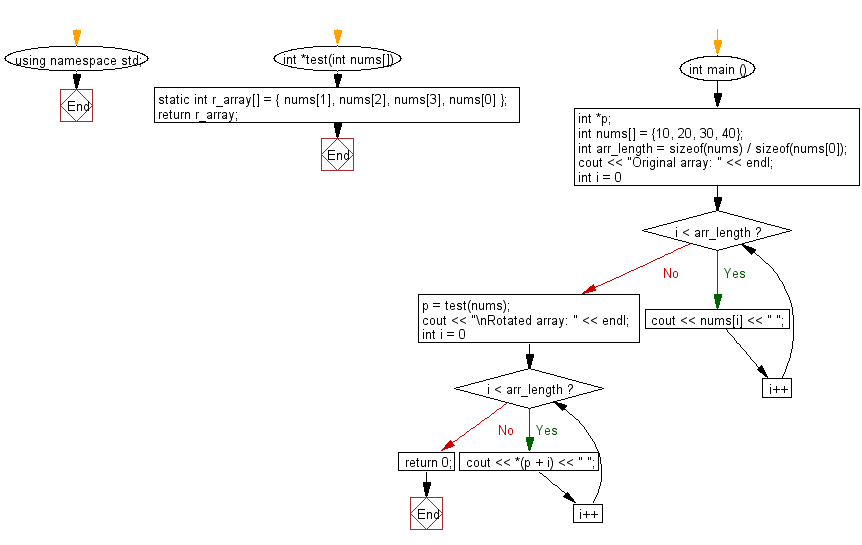
For more Practice: Solve these Related Problems:
- Write a C++ program to perform a left rotation on an array of exactly 4 integers and output the rotated array.
- Write a C++ program that reads 4 integers into an array, rotates the array left by one position, and prints the result.
- Write a C++ program to cyclically shift the elements of a 4-element array to the left and display the new order.
- Write a C++ program that rotates a fixed-length (4) array leftwards by moving the first element to the end.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to compute the sum of the elements of a given array of integers.
Next: Write a C++ program to reverse a given array of integers and length 5.
What is the difficulty level of this exercise?