C++ Exercises: Reverse a given array of integers and length 5
Reverse Array (Length 5)
Write a C++ program to reverse a given array of integers of length 5.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that creates and returns a reversed array
int *test(int nums[]) {
// Static array storing the elements of the input array in reverse order
static int r_array[] = {nums[4], nums[3], nums[2], nums[1], nums[0] };
return r_array; // Returning the reversed array
}
// Main function
int main () {
int *p; // Pointer to an integer
int nums[] = {0, 10, 20, 30, 40}; // Define an array nums
int arr_length = sizeof(nums) / sizeof(nums[0]); // Calculate the length of nums
cout << "Original array: " << endl;
for ( int i = 0; i < arr_length; i++ ) {
cout << nums[i] << " "; // Output the original array
}
p = test(nums); // Reverse the array and assign it to pointer p
cout << "\nReverse array: " << endl;
for ( int i = 0; i < arr_length; i++ ) {
cout << *(p + i) << " "; // Output the reversed array
}
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
Original array: 0 10 20 30 40 Reverse array: 40 30 20 10 0
Visual Presentation:
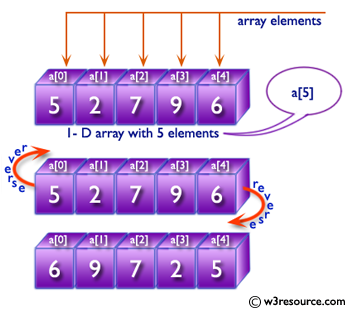
Flowchart:
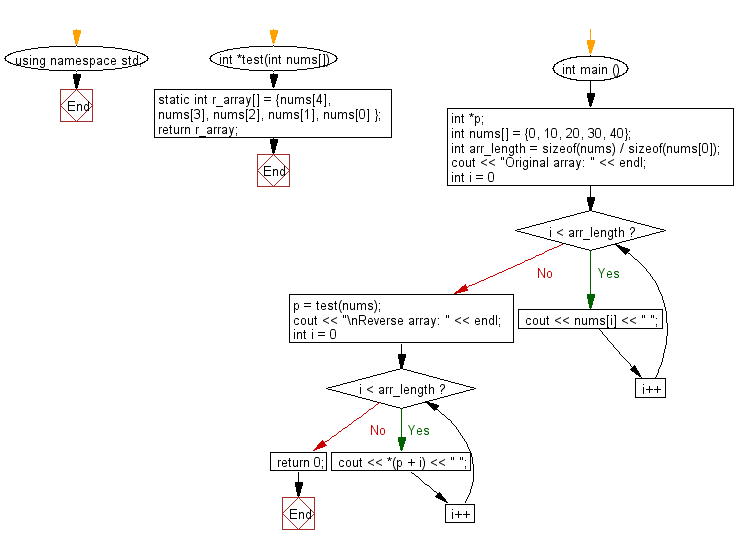
For more Practice: Solve these Related Problems:
- Write a C++ program to reverse the order of a 5-element integer array and print the reversed array.
- Write a C++ program that reads exactly 5 integers, reverses their order, and outputs the result using a loop.
- Write a C++ program to take a 5-element array as input, reverse its elements, and then print the new sequence.
- Write a C++ program that implements array reversal for a fixed size of 5 and displays the array in reverse order.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to rotate the elements of a given array of integers (length 4 ) in left direction and return the new array.
Next: Write a C++ program to create a new array containing the middle elements from the two given arrays of integers, each length 5.
What is the difficulty level of this exercise?