C++ Exercises: Create a new array containing the middle elements from the two given arrays of integers, each length 5
Middle Elements from Two Arrays
Write a C++ program to create an array containing the middle elements from the two given arrays of integers, each of length 5.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that creates and returns an array containing elements from given indices of two input arrays
int *test(int nums1[], int nums2[]) {
// Static array storing the elements at index 2 from nums1 and nums2
static int max_array[] = { nums1[2], nums2[2] };
return max_array; // Returning the new array
}
// Main function
int main () {
int *p; // Pointer to an integer
int nums1[] = {0, 10, 20, 30, 40}; // Define an array nums1
int nums2[] = {0, -10, -20, -30, -40}; // Define an array nums2
p = test(nums1, nums2); // Store the result of test function in pointer p
cout << "\nNew array: " << endl;
for ( int i = 0; i < 2; i++ ) {
cout << *(p + i) << " "; // Output the new array elements
}
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
New array: 20 -20
Visual Presentation:
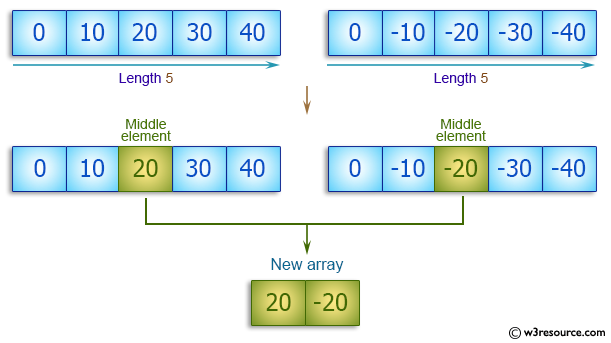
Flowchart:
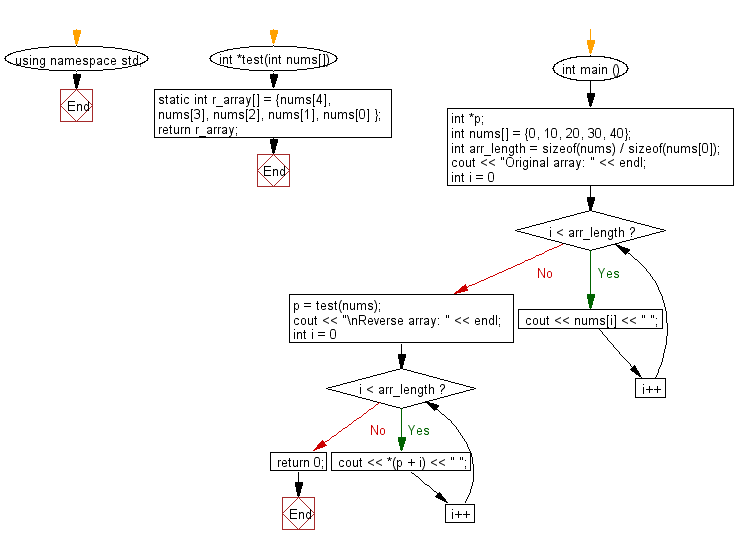
For more Practice: Solve these Related Problems:
- Write a C++ program to extract the middle element from two separate 5-element arrays and combine them into a new array.
- Write a C++ program that reads two arrays of length 5 and outputs an array containing the middle element of each.
- Write a C++ program to obtain the central elements of two given 5-element arrays and print them as a new 2-element array.
- Write a C++ program that processes two arrays of fixed length 5 and creates a new array by merging their median elements.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to reverse a given array of integers and length 5.
Next: Write a C++ program to create a new array taking the first and last elements of a given array of integers and length 1 or more.
What is the difficulty level of this exercise?