C++ Exercises: Create a new array taking the first and last elements of a given array of integers and length 1 or more
First and Last Elements of Array
Write a C++ program to create an array taking the first and last elements of a given array of integers and length 1 or more.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that creates and returns an array containing the first and last elements of the input array
int *test(int nums[], int arr_length) {
// Static array storing the first and last elements of the nums array
static int r_array[] = {nums[0], nums[arr_length - 1] };
return r_array; // Returning the new array
}
// Main function
int main () {
int *p; // Pointer to an integer
int nums[] = { 10, 20, -30, -40, 30 }; // Define an array nums
int arr_length = sizeof(nums) / sizeof(nums[0]); // Calculate the length of nums
cout << "Original array: " << endl;
for ( int i = 0; i < arr_length; i++ ) {
cout << nums[i] << " "; // Output the original array
}
p = test(nums, arr_length); // Store the result of test function in pointer p
arr_length = sizeof(p) / sizeof(p[0]); // Calculate the length of p (which won't work correctly)
cout << "\nNew array: " << endl;
for ( int i = 0; i < arr_length; i++ ) {
cout << *(p + i) << " "; // Output the new array elements (this size calculation won't work correctly)
}
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
Original array: 10 20 -30 -40 30 New array: 10 30
Visual Presentation:
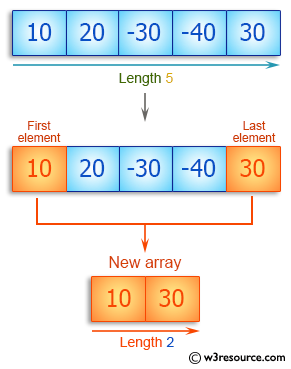
Flowchart:
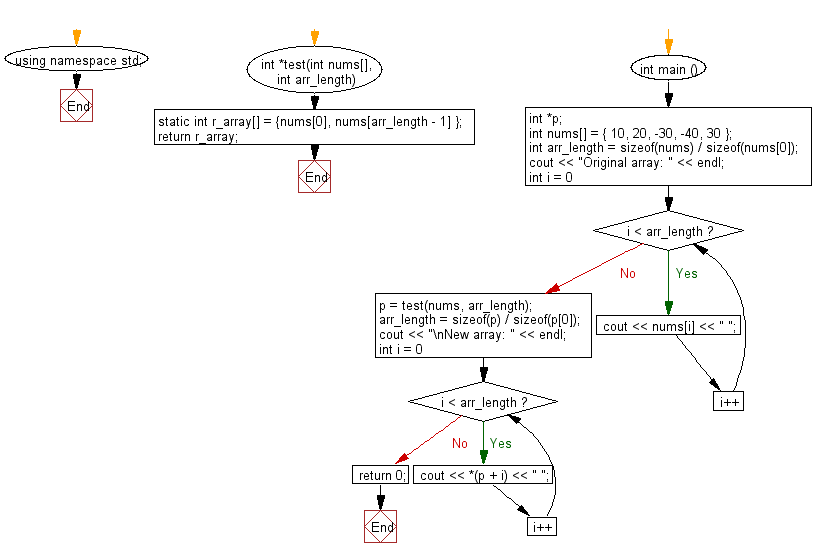
For more Practice: Solve these Related Problems:
- Write a C++ program to construct a new array consisting of the first and last elements of a given integer array.
- Write a C++ program that reads an array and outputs a 2-element array containing its first and last values.
- Write a C++ program to extract and print the boundary elements of an input array, forming a new array with them.
- Write a C++ program that takes an integer array and creates a new array by combining its first and last elements.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new array containing the middle elements from the two given arrays of integers, each length 5.
Next: Write a C++ program to check if a given array of integers and length 2, contains 15 or 20.
What is the difficulty level of this exercise?