C++ Exercises: Check if a given array of integers and length 2, contains 15 or 20
Check 15 or 20 in Array (Length 2)
Write a C++ program to determine whether a given array of integers of length 2 contains 15 or 20.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that checks if any of the first two elements in the array are equal to 15 or 20
bool test(int nums[]) {
return nums[0] == 15 || nums[0] == 20 || nums[1] == 15 || nums[1] == 20;
}
// Main function
int main () {
int nums1[] = { 12, 20 }; // Define an array nums1
int nums2[] = { 14, 15 }; // Define an array nums2
int nums3[] = { 11, 21 }; // Define an array nums3
// Output the result of test function for nums1, nums2, and nums3 arrays
cout << test(nums1) << endl;
cout << test(nums2) << endl;
cout << test(nums3) << endl;
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
1 1 0
Visual Presentation:
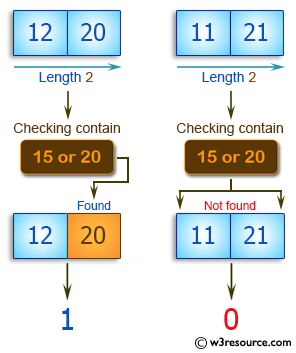
Flowchart:
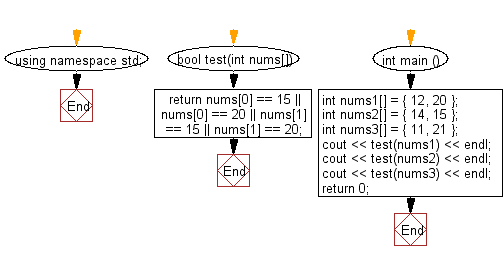
For more Practice: Solve these Related Problems:
- Write a C++ program to determine whether a 2-element integer array contains either 15 or 20, returning a boolean result.
- Write a C++ program that reads two integers and checks if at least one is equal to 15 or 20, then outputs true if found.
- Write a C++ program to check a 2-element array for the presence of the numbers 15 or 20 and print 1 if either exists.
- Write a C++ program that validates a two-integer array by returning true if one of the elements is 15 or 20, otherwise false.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new array taking the first and last elements of a given array of integers and length 1 or more.
Next: Write a C++ program to check if a given array of integers and length 2, does not contain 15 or 20.
What is the difficulty level of this exercise?