C++ Exercises: Check if a given array of integers and length 2, does not contain 15 or 20
Check if 15 or 20 Not Present in Array (Length 2)
Write a C++ program to check if an array of integers with length 2 does not contain 15 or 20.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that checks if neither of the first two elements in the array are equal to 15 or 20
bool test(int nums[]) {
return nums[0] != 15 && nums[0] != 20 && nums[1] != 15 && nums[1] != 20;
}
// Main function
int main () {
int nums1[] = { 12, 20 }; // Define an array nums1
int nums2[] = { 14, 15 }; // Define an array nums2
int nums3[] = { 11, 21 }; // Define an array nums3
// Output the result of test function for nums1, nums2, and nums3 arrays
cout << test(nums1) << endl;
cout << test(nums2) << endl;
cout << test(nums3) << endl;
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
0 0 1
Visual Presentation:
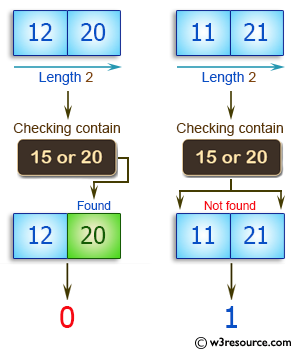
Flowchart:
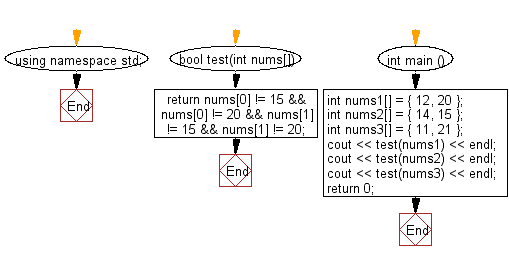
For more Practice: Solve these Related Problems:
- Write a C++ program to check a 2-element array and return true if neither element is 15 or 20.
- Write a C++ program that reads two integers and outputs false if either is 15 or 20, and true if neither is present.
- Write a C++ program to determine if a 2-element array does not contain 15 or 20, printing the corresponding boolean.
- Write a C++ program that checks whether both elements of a two-integer array differ from 15 and 20, then returns true if so.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if a given array of integers and length 2, contains 15 or 20.
Next: Write a C++ program to check a given array of integers and return true if the array contains 10 or 20 twice. The length of the array will be 0, 1, or 2.
What is the difficulty level of this exercise?