C++ Exercises: Create an array taking two middle elements from a given array of integers of length even
Create Array from Middle Elements of Even-Length Array
Write a C++ program to create an array taking two middle elements from a given array of integers of length even.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
static int *test(int numbers[], int arr_length) // Function definition that returns a pointer to an array
{
static int r_array[] = { numbers[arr_length / 2 - 1], numbers[arr_length / 2] }; // Creating a new array with elements from the middle of 'numbers' array
return r_array; // Returning a pointer to the newly created array
}
int main () {
int *p, i; // Declaring pointer variable p and integer i
int nums1[] = { 1, 5, 7, 9, 11, 13 }; // Initializing array nums1 with values
cout << "Original array:" << endl;
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
for ( i = 0; i < arr_length; i++ ) { // Loop to print elements of the nums1 array
cout << nums1[i] << " "; // Printing elements of the nums1 array
}
p = test(nums1, arr_length); // Calling the test function and storing the returned pointer in p
cout << "\nNew array: " << endl; // Printing a message
arr_length = sizeof(p) / sizeof(p[0]); // Recalculating length of array pointed by p (This may not work as intended, see explanation below)
for ( i = 0; i < arr_length; i++ ) { // Loop to print elements of the array pointed by p
cout << *(p + i) << " "; // Printing elements of the array pointed by p
}
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
Original array: 1 5 7 9 11 13 New array: 7 9
Visual Presentation:
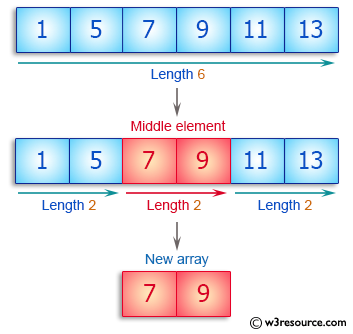
Flowchart:
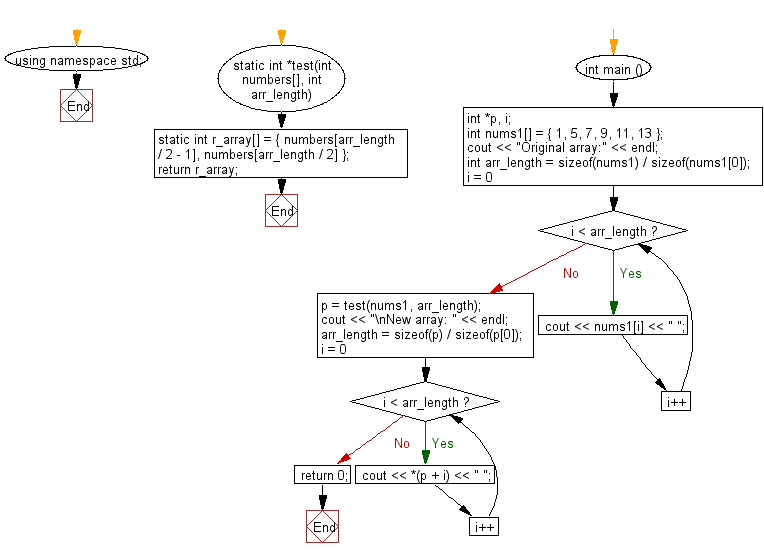
For more Practice: Solve these Related Problems:
- Write a C++ program to extract the two central elements from an even-length array and create a new array containing them.
- Write a C++ program that reads an array of even length and outputs a new array composed solely of its middle two numbers.
- Write a C++ program to process an even-length array and form a new array with the elements located at the middle positions.
- Write a C++ program that computes the midpoint of an even-length array and returns an array of the two middle values.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array of integers, length 3 and create a new array. If there is a 5 in the given array immediately followed by a 7 then set 7 to 1.
Next: Write a C++ program to create a new array swapping the first and last elements of a given array of integers and length will be least 1.
What is the difficulty level of this exercise?