C++ Exercises: Create a new array swapping the first and last elements of a given array of integers and length will be least 1
Swap First and Last Elements of Array
Write a C++ program to create an array by swapping the first and last elements of a given array of integers with a length of at least 1.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
static int *test(int numbers[], int arr_length) // Function definition that swaps the first and last elements of an array
{
int first = numbers[0]; // Storing the first element of the array
numbers[0] = numbers[arr_length - 1]; // Swapping the first element with the last element of the array
numbers[arr_length - 1] = first; // Assigning the stored first element to the last position
return numbers; // Returning a pointer to the modified array
}
int main () {
int *p, i; // Declaring pointer variable p and integer i
int nums1[] = { 1, 5, 7, 9, 11, 13 }; // Initializing array nums1 with values
cout << "Original array:" << endl;
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
for ( i = 0; i < arr_length; i++ ) { // Loop to print elements of the nums1 array
cout << nums1[i] << " "; // Printing elements of the nums1 array
}
p = test(nums1, arr_length); // Calling the test function and storing the returned pointer in p
cout << "\nNew array (after swapping the first and last elements of the said array): " << endl; // Printing a message
for ( i = 0; i < arr_length; i++ ) { // Loop to print elements of the array pointed by p
cout << *(p + i) << " "; // Printing elements of the array pointed by p
}
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
Original array: 1 5 7 9 11 13 New array (after swapping the first and last elements of the said array): 13 5 7 9 11 1
Visual Presentation:
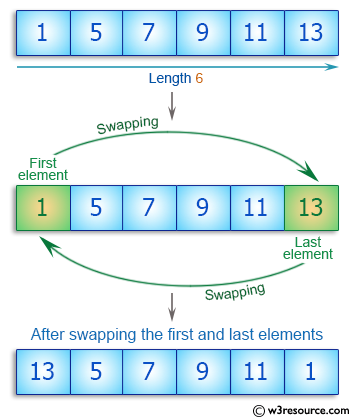
Flowchart:
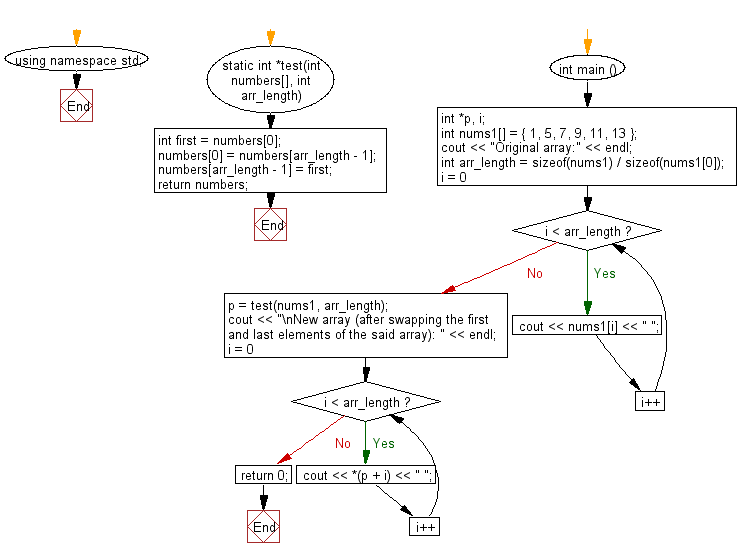
For more Practice: Solve these Related Problems:
- Write a C++ program to swap the first and last elements of an integer array of any length and print the modified array.
- Write a C++ program that reads an array and interchanges its first and last items, then outputs the resulting array.
- Write a C++ program to create a new array by exchanging the first and last elements of a given array, keeping all other elements unchanged.
- Write a C++ program that processes an input array and swaps its boundary elements, printing the new configuration.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create an array taking two middle elements from a given array of integers of length even.
Next: Write a C++ program to create a new array length 3 from a given array (length atleast 3) using the elements from the middle of the array.
What is the difficulty level of this exercise?