C++ Exercises: Find the largest value from first, last, and middle elements of a given array of integers of odd length
Find Largest of First, Last, Middle Elements in Odd-Length Array
Write a C++ program to find the largest value from the first, last, and middle elements of a given array of integers of odd length (at least 1).
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that finds the maximum value among the first, middle, and last elements of an array
static int test(int numbers[], int arr_length)
{
int first, middle_ele, last_ele, max_ele; // Declaring integer variables
first = numbers[0]; // Storing the first element of the array
middle_ele = numbers[arr_length / 2]; // Storing the middle element of the array
last_ele = numbers[arr_length - 1]; // Storing the last element of the array
max_ele = first; // Initializing max_ele with the first element of the array
// Checking if middle element is greater than max_ele
if (middle_ele > max_ele)
{
max_ele = middle_ele; // Updating max_ele if middle element is greater
}
// Checking if last element is greater than max_ele
if (last_ele > max_ele)
{
max_ele = last_ele; // Updating max_ele if last element is greater
}
return max_ele; // Returning the maximum element among first, middle, and last
}
int main()
{
int nums1[] = {1}; // Initializing array nums1 with a single element
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
// Calling test function with nums1 and displaying the result
cout << test(nums1, arr_length) << endl;
int nums2[] = {1,2,9}; // Initializing array nums2 with three elements
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculating length of array nums2
// Calling test function with nums2 and displaying the result
cout << test(nums2, arr_length) << endl;
int nums3[] = {1,2,9,3,3}; // Initializing array nums3 with five elements
cout << test(nums3, arr_length) << endl; // Calling test function with nums3 and displaying the result
int nums4[] = {1,2,3,4,5,6,7}; // Initializing array nums4 with seven elements
arr_length = sizeof(nums4) / sizeof(nums4[0]); // Calculating length of array nums4
// Calling test function with nums4 and displaying the result
cout << test(nums4, arr_length) << endl;
int nums5[] = {1,2,2,3,7,8,9,10,6,5,4}; // Initializing array nums5 with eleven elements
arr_length = sizeof(nums5) / sizeof(nums5[0]); // Calculating length of array nums5
// Calling test function with nums5 and displaying the result
cout << test(nums5, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
1 9 9 7 8
Visual Presentation:
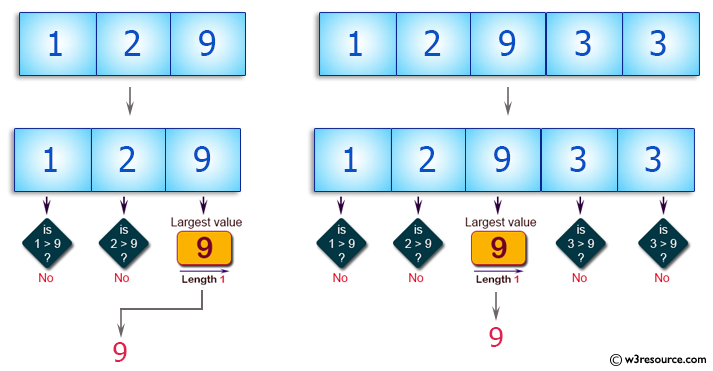
Flowchart:
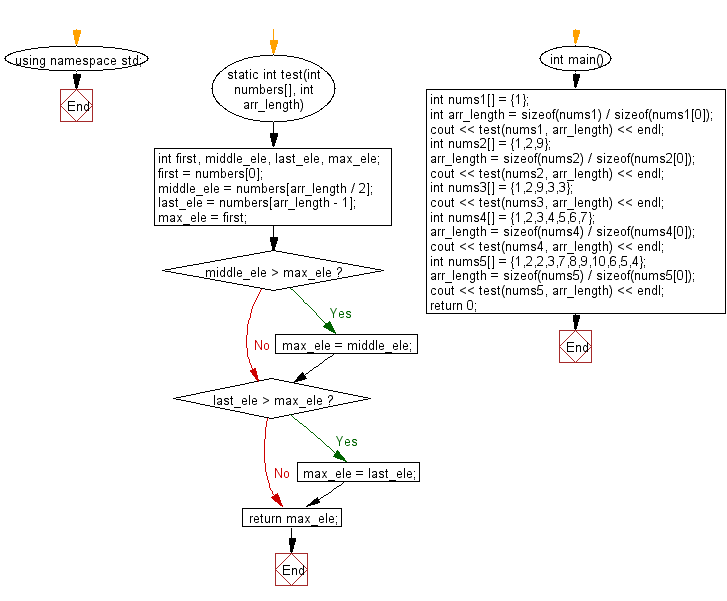
For more Practice: Solve these Related Problems:
- Write a C++ program to compare the first, middle, and last elements of an odd-length array and print the largest value.
- Write a C++ program that reads an odd-length array and outputs the maximum value among its first, central, and final elements.
- Write a C++ program to determine the greatest number among the boundary and middle element of an odd-sized array.
- Write a C++ program that examines an odd-length integer array and returns the highest value from the first, middle, and last positions.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new array length 3 from a given array (length atleast 3) using the elements from the middle of the array.
Next: Write a C++ program to count even number of elements in a given array of integers.
What is the difficulty level of this exercise?