C++ Exercises: Count even number of elements in a given array of integers
Count Even Numbers in Array
Write a C++ program to count the even number of elements in a given array of integers.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that counts the number of even integers in the input array
static int test(int nums[], int arr_length)
{
int evens = 0; // Initializing a counter for even numbers
// Loop through the array to count even numbers
for (int i = 0; i < arr_length; i++)
{
if (nums[i] % 2 == 0) // Checking if the element is even
evens++; // Incrementing the counter if the number is even
}
return evens; // Returning the count of even numbers
}
int main()
{
int nums1[] = {1, 5, 7, 9, 10, 12}; // Initializing array nums1 with various integers
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
// Calling test function with nums1 and displaying the count of even numbers
cout << test(nums1, arr_length) << endl;
int nums2[] = {0, 2, 4, 6, 8, 10}; // Initializing array nums2 with even integers
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculating length of array nums2
// Calling test function with nums2 and displaying the count of even numbers
cout << test(nums2, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
2 6
Visual Presentation:
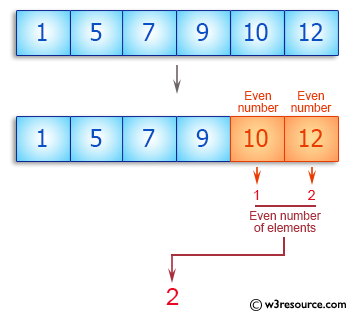
Flowchart:
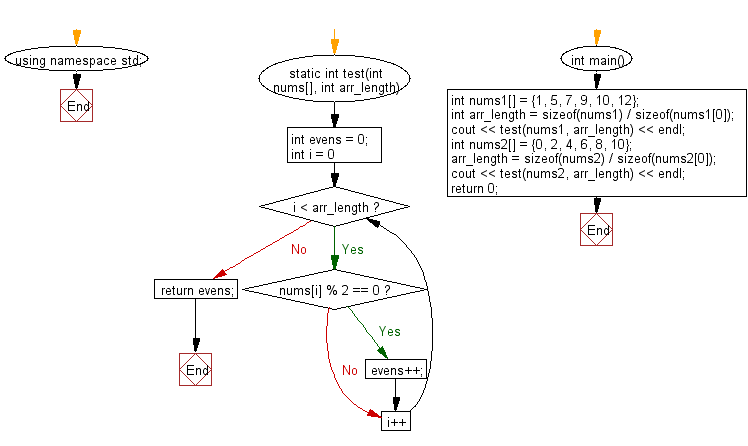
For more Practice: Solve these Related Problems:
- Write a C++ program to count the number of even integers in an array and output the total count.
- Write a C++ program that iterates over an array of integers and prints the count of elements that are even.
- Write a C++ program to process an input array and return the number of even numbers, using modulus for determination.
- Write a C++ program that reads an integer array and computes the frequency of even values, then displays the result.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the largest value from first, last, and middle elements of a given array of integers of odd length (atleast 1).
Next: Write a C++ program to compute the difference between the largest and smallest values in a given array of integers and length one or more.
What is the difficulty level of this exercise?