C++ Exercises: Read seven numbers and sorts them in descending order
Sort Seven Numbers in Descending
Write a C++ program that reads seven numbers and sorts them in descending order.
Visual Presentation:
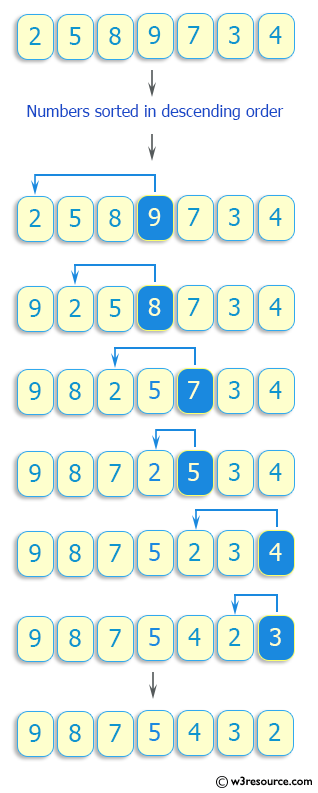
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using the standard namespace
int main() {
int num[7]; // Declaring an array 'num' of size 7 to store integers
// Loop to read 7 integers from the input and store them in the array 'num'
for (int i = 0; i != 7; ++i) {
cin >> num[i]; // Reading integers from the input and storing them in 'num'
}
sort(num, num+7); // Sorting the elements in the 'num' array in ascending order
// Outputting the elements of 'num' array in descending order (from largest to smallest)
cout << " " << num[6] << " " << num[5] << " " << num[4] << " " << num[3] << " " << num[2] << " " << num[1] << " " << num[0];
return 0; // Indicating successful completion of the program
}
Sample Output:
Sample Input 2 5 8 9 7 3 4 sample Output 9 8 7 5 4 3 2
Flowchart:
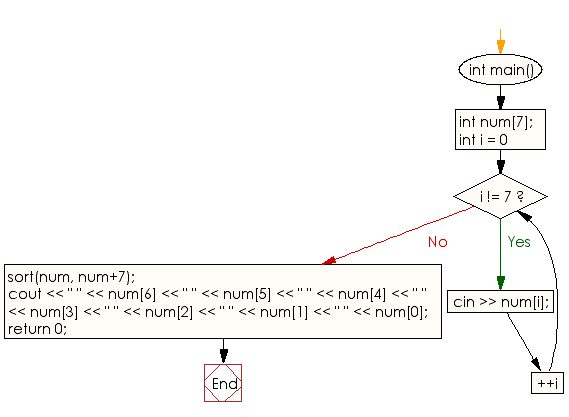
For more Practice: Solve these Related Problems:
- Write a C++ program to sort seven numbers in descending order using the bubble sort algorithm without using extra arrays.
- Write a C++ program that reads seven numbers and sorts them in descending order using recursion.
- Write a C++ program to sort a fixed-size array of seven numbers in descending order and then print the sorted array with indices.
- Write a C++ program that sorts seven numbers in descending order using a selection sort algorithm and displays intermediate steps.
C++ Code Editor:
Previous: Write a C++ program to which prints the central coordinate and the radius of a circumscribed circle of a triangle which is created by three points on the plane surface.
Next: Write a C++ program to read an integer n and prints the factorial of n, assume that n ≤ 10.
What is the difficulty level of this exercise?