C++ Exercises: Read an integer n and prints the factorial of n
Factorial of n
For n = 10, write a C++ program that reads the integer n and prints its factorial.
Visual Presentation:
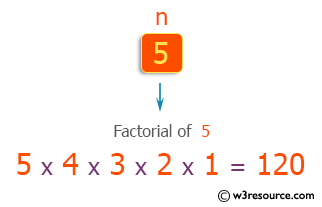
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using the standard namespace
// Function to calculate factorial recursively
long long factorial(int num) {
if (num == 0) { // If the number is 0
return 1; // Return 1 because 0! is 1 by definition
}
else {
// Recursive call to calculate factorial
// Multiplies the number with the factorial of (number - 1)
return num * factorial(num - 1);
}
}
int main() {
int num; // Declare variable to store the input number
cin >> num; // Take input from the user
// Displaying the factorial of the input number using the factorial function
cout << factorial(num) << endl;
return 0; // Indicating successful completion of the program
}
Sample Output:
sample Output 1
Flowchart:
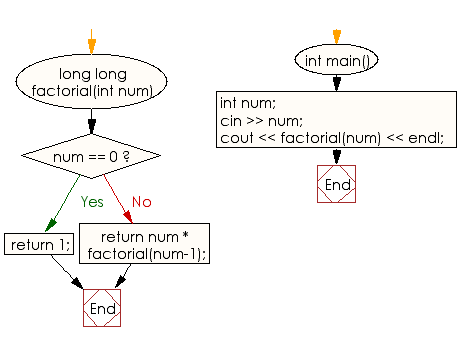
For more Practice: Solve these Related Problems:
- Write a C++ program to calculate the factorial of n using both recursion and iteration, then compare the results.
- Write a C++ program to compute the factorial of n while checking for integer overflow with large values.
- Write a C++ program that calculates n! using a loop and prints the multiplication sequence leading to the final result.
- Write a C++ program to compute the factorial of n using tail recursion and then display the number of digits in the result.
C++ Code Editor:
Previous: Write a C++ program to read seven numbers and sorts them in descending order.
Next: Write a C++ program to replace all the lower-case letters of a given string with the corresponding capital letters.
What is the difficulty level of this exercise?