C++ Exercises: Add up all the digits between two numbers
C++ Basic: Exercise-86 with Solution
Write a C++ program to add up all the digits between two given integers.
Add all the digits between 11 and 16.
// 11, 12, 13, 14, 15, 16
(1 + 1) + (1 +2) + (1 + 3) + (1 + 4) + (1 + 5) + (1 + 6)
= 2 + 3 + 4 + 5 + 6 + 7
= 27
Sample Data:
(5, 9) -> 35
(12, 18) -> 42
(39, 41) -> 21
Visual Presentation:
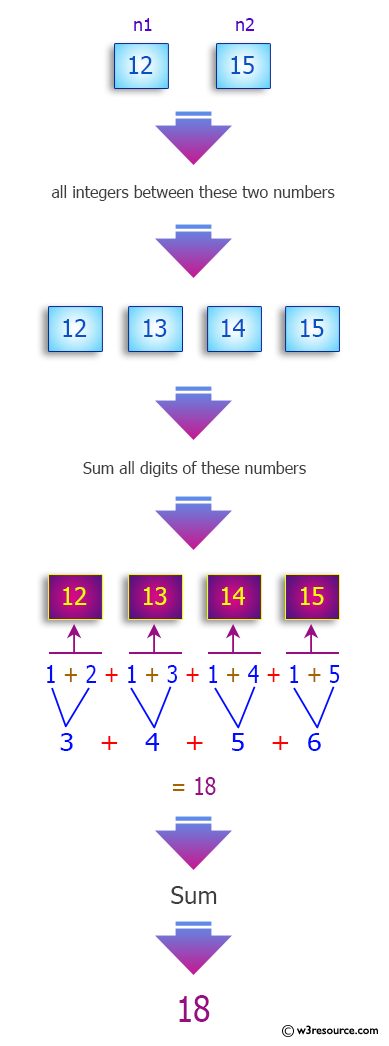
Sample Solution-1:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' calculates the sum of digits for all numbers between x and y (inclusive)
int test(int x, int y)
{
int digit_sum = 0;
for(int i = x; i <= y; ++i)
{
int p = i;
// Loop to extract digits and calculate their sum for each number between x and y
while(p > 0)
{
digit_sum += p % 10; // Extract the last digit of the number and add it to digit_sum
p /= 10; // Remove the last digit from the number
}
}
return digit_sum; // Return the total sum of digits between x and y
}
int main() {
// Define the range of numbers to calculate the sum of their digits
int n1 = 39;
int n2 = 41;
// Display the range of numbers and the sum of their digits using the 'test' function
cout << "Add up all the digits between " << n1 << " and " << n2 << " is: ";
cout << test(n1, n2) << endl;
}
Sample Output:
Add up all the digits between 39 and 41 is: 21
Flowchart:
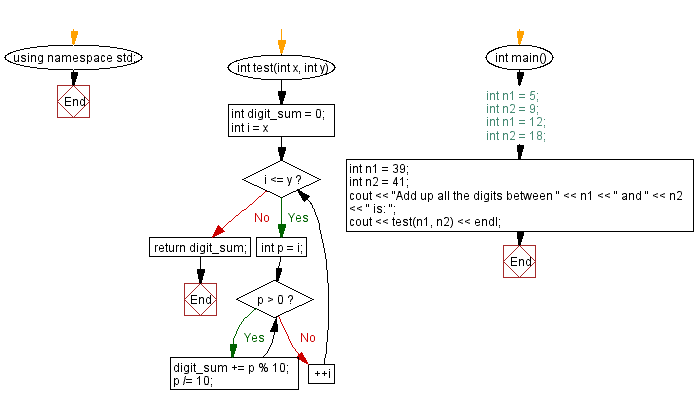
Sample Solution-2:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' calculates the sum of digits for all numbers between x and y (inclusive)
int test(int x, int y)
{
int digit_sum = 0; // Initialize the variable to store the sum of digits
int t = x; // Create a temporary variable to iterate from x to y
while (t <= y) { // Loop through the numbers from x to y (inclusive)
x = t; // Set x to the current value of t
while (x) { // Loop to extract digits and calculate their sum for each number between x and y
digit_sum += x % 10; // Extract the last digit of 'x' and add it to digit_sum
x /= 10; // Remove the last digit from 'x'
}
++t; // Move to the next number in the range
}
return digit_sum; // Return the total sum of digits between x and y
}
int main() {
// Define the range of numbers to calculate the sum of their digits
int n1 = 39;
int n2 = 41;
// Display the range of numbers and the sum of their digits using the 'test' function
cout << "Add up all the digits between " << n1 << " and " << n2 << " is: ";
cout << test(n1, n2) << endl;
}
Sample Output:
Add up all the digits between 39 and 41 is: 21
Flowchart:
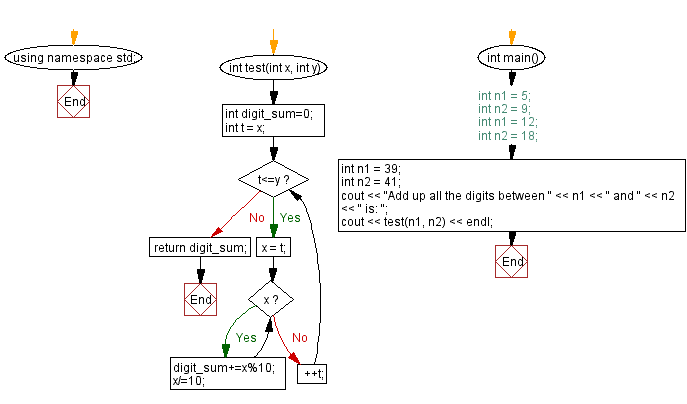
C++ Code Editor:
Previous C++ Exercise: Find the total number of minutes between two times.
Next C++ Exercises: C++ For Loop Exercises Home.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics