C++ Exercises: Find the total number of minutes between two given times
C++ Basic: Exercise-85 with Solution
Minutes Between Two Times
Write a C++ program to find the total number of minutes between two given times (formatted with a colon and am or pm).
Visual Presentation:
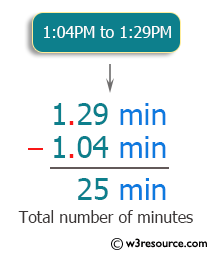
Sample Solution:
C++ Code :
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
// Function to calculate the difference in minutes between two times
int count_minutes(string time_str) {
// Variables to store time components
string num1, num2;
string hr1, hr2, min1, min2;
int index = time_str.find('-');
bool colon = false;
// Iterating through the time string to extract hour and minute values
for (int x = 0; x < time_str.length(); x++) {
if (x >= index) {
colon = false;
for (x; x < time_str.length(); x++) {
if (time_str[x] == ':') {
colon = true;
continue;
}
if (time_str[x] == 'A' || time_str[x] == 'P') {
num2.push_back(time_str[x]);
num2.push_back(time_str[x + 1]);
}
if (colon && isdigit(time_str[x])) {
min2.push_back(time_str[x]);
} else if (isdigit(time_str[x])) {
hr2.push_back(time_str[x]);
}
}
} else {
if (time_str[x] == ':') {
colon = true;
continue;
}
if (time_str[x] == 'A' || time_str[x] == 'P') {
num1.push_back(time_str[x]);
num1.push_back(time_str[x + 1]);
}
if (colon && isdigit(time_str[x])) {
min1.push_back(time_str[x]);
} else if (isdigit(time_str[x])) {
hr1.push_back(time_str[x]);
}
}
}
// Calculating the hour difference
int hr = 0;
if (stoi(hr1) == stoi(hr2) && num1 == num2 && stoi(min1) > stoi(min2)) {
hr = 24 - (stoi(hr1) - stoi(hr2));
} else if (stoi(hr1) > stoi(hr2) && num1 == num2 && stoi(min1) < stoi(min2)) {
hr = 24 - (stoi(hr1) - stoi(hr2));
} else if (num1 == num2 || (hr2 == "12" && hr1 != "12")) {
hr = stoi(hr2) - stoi(hr1);
} else {
hr = (12 - stoi(hr1)) + stoi(hr2);
}
// Calculating the total time difference in minutes
int time;
if (min1 != "00") {
time = (hr * 60 - stoi(min1)) + stoi(min2);
} else {
time = (hr * 60 + stoi(min1)) + stoi(min2);
}
return time;
}
// Main function to test the count_minutes function with different time inputs
int main() {
cout << "Minutes between 12:01AM to 12:00PM: "<< count_minutes("12:01PM-12:00PM") << endl;
cout << "Minutes between 2:12AM to 2:8AM: "<< count_minutes("2:12AM-2:8AM") << endl;
cout << "Minutes between 1:04PM to 1:29PM: "<< count_minutes("1:04PM-1:29PM") << endl;
cout << "Minutes between 2:00PM to 10:00PM: "<< count_minutes("2:00PM-10:00PM") << endl;
return 0;
}
Sample Output:
Minutes between 12:01AM to 12:00PM: 1439 Minutes between 2:12AM to 2:8AM: 1436 Minutes between 1:04PM to 1:29PM: 25 Minutes between 2:00PM to 10:00PM: 480
Flowchart:
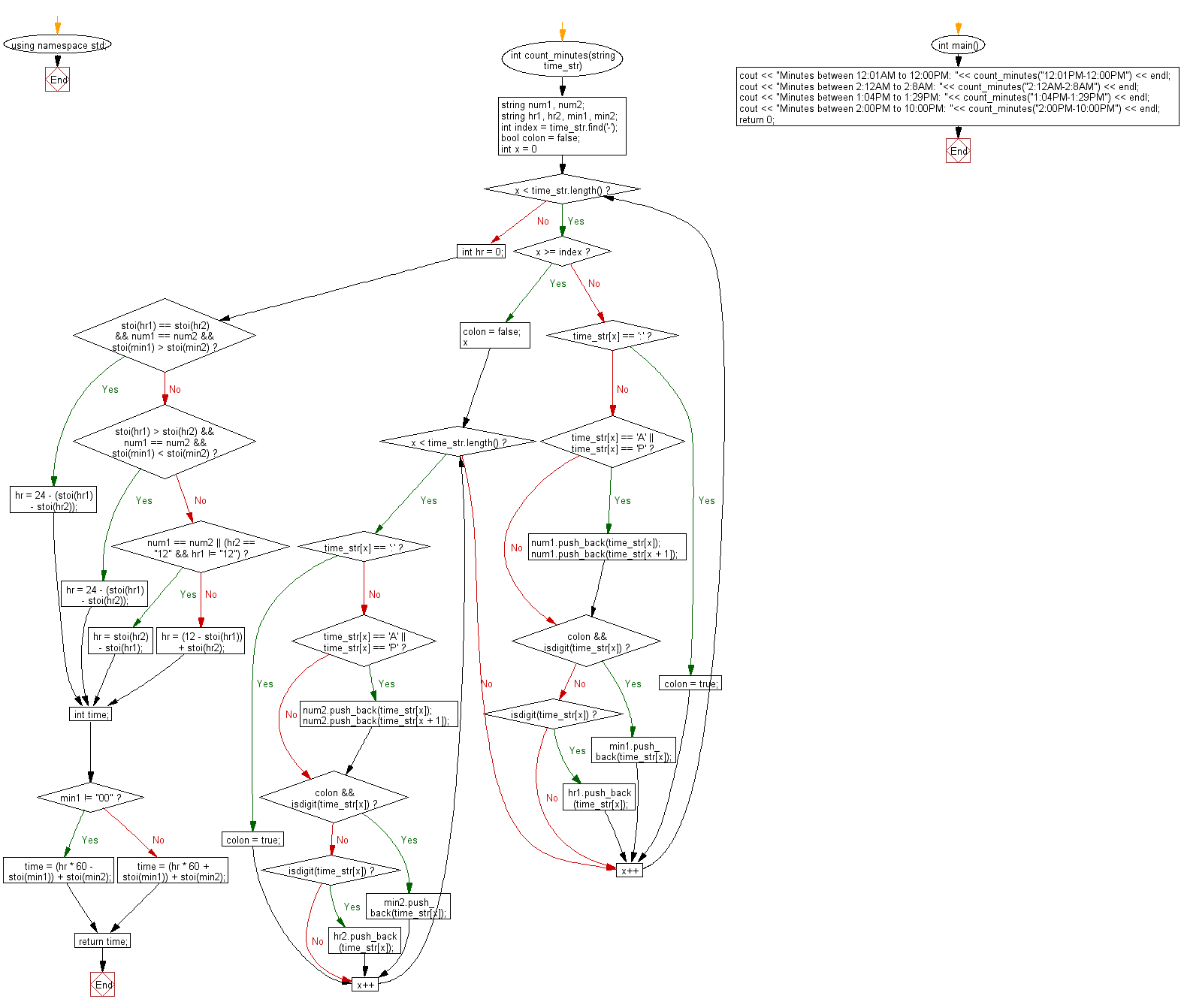
C++ Code Editor:
Previous C++ Exercise: Convert a given number into hours and minutes.
Next C++ Exercise: Add up all the digits between two numbers .
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/basic/cpp-basic-exercise-85.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics