C++ Exercises: Find the sum of the series 1 + 1/2^2 + 1/3^3 + …..+ 1/n^n
C++ For Loop: Exercise-11 with Solution
Write a program in C++ to find the sum of the series 1 + 1/2^2 + 1/3^3 + …..+ 1/n^n.
Visual Presentation:
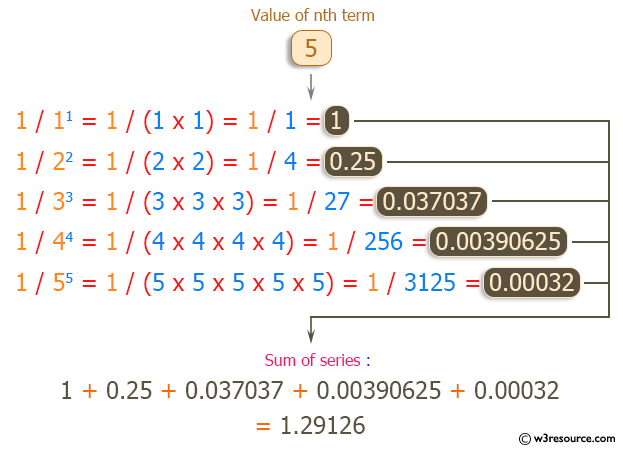
Sample Solution :-
C++ Code :
#include <iostream> // Including the input/output stream header file
#include <math.h> // Including the math library header file
using namespace std; // Using the standard namespace to avoid writing std::
int main() // Start of the main function
{
double sum = 0, a; // Declaration of double variables 'sum' and 'a'
int n, i; // Declaration of integer variables 'n' and 'i'
// Display a message to find the sum of the series 1 + 1/2^2 + 1/3^3 +...+ 1/n^n
cout << "\n\n Find the sum of the series 1 + 1/2^2 + 1/3^3 +.....+ 1/n^n:\n";
cout << "----------------------------------------------------------------\n";
// Prompt the user to input the value for the nth term of the series
cout << " Input the value for nth term: ";
cin >> n; // Read the value entered by the user
for (i = 1; i <= n; ++i) // Loop to calculate each term of the series
{
a = 1 / pow(i, i); // Calculate the current term: 1/(i^i)
cout << "1/" << i << "^" << i << " = " << a << endl; // Display the current term
sum += a; // Add the current term to the sum
}
// Display the total sum of the series
cout << " The sum of the above series is: " << sum << endl;
return 0; // Indicating successful completion of the program
}
Sample Output:
Find the sum of the series 1 + 1/2^2 + 1/3^3 +.....+ 1/n^n: ---------------------------------------------------------------- Input the value for nth term: 5 1/1^1 = 1 1/2^2 = 0.25 1/3^3 = 0.037037 1/4^4 = 0.00390625 1/5^5 = 0.00032 The sum of the above series is: 1.29126
Flowchart:
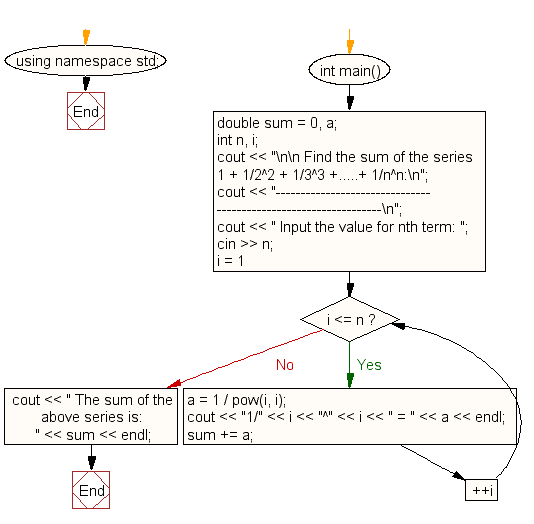
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the sum of digits of a given number.
Next: Write a program in C++ to calculate the sum of the series (1*1) + (2*2) + (3*3) + (4*4) + (5*5) + ... + (n*n).
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/for-loop/cpp-for-loop-exercise-11.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics