C++ Exercises: List non-prime numbers from 1 to an upperbound
16. List Non-Prime Numbers from 1 to Upper Bound
Write a C++ program to list non-prime numbers from 1 to an upperbound.
Visual Presentation:
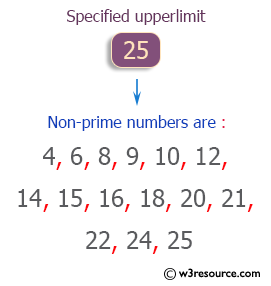
Sample Solution :-
C++ Code :
#include <iostream> // Include input-output stream header
#include <cmath> // Include math functions header
using namespace std; // Using standard namespace to avoid writing std::
int main() // Start of the main function
{
int ult; // Declaration of variable 'ult' to store the upper limit
cout << "\n\n List non-prime numbers from 1 to an upperbound:\n"; // Display message on the console
cout << "----------------------------------------------------\n";
cout << " Input the upperlimit: "; // Prompt the user to input the upper limit
cin >> ult; // Read the input value as the upper limit
cout << " The non-prime numbers are: " << endl; // Display message for non-prime numbers
// Loop through numbers from 2 to the upper limit to check for non-prime numbers
for (int num = 2; num <= ult; ++num)
{
int mfactor = (int)sqrt(num); // Find the square root of the number and cast it to an integer as the maximum factor to check
// Loop to check for factors of the current number from 2 to its square root
for (int fact = 2; fact <= mfactor; ++fact)
{
// If the current number is divisible by 'fact', it's not a prime number
if (num % fact == 0)
{
cout << num << " "; // Display the non-prime number
break; // Break the loop to move to the next number
}
}
}
cout << endl; // Output a newline
return 0; // Indicates successful termination of the program
}
Sample Output:
List non-prime numbers from 1 to an upperbound: ---------------------------------------------------- Input the upperlimit: 25 The non-prime numbers are: 4 6 8 9 10 12 14 15 16 18 20 21 22 24 25
Flowchart:
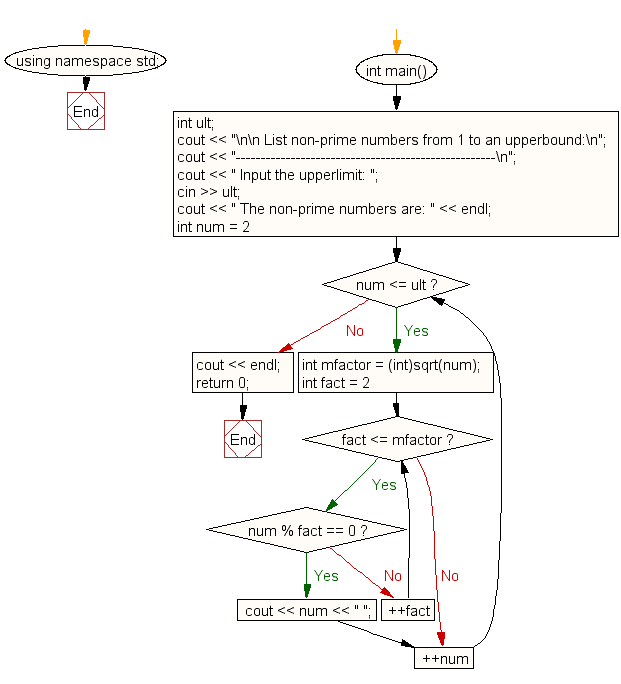
For more Practice: Solve these Related Problems:
- Write a C++ program to display all composite numbers between 1 and a user-specified upper limit.
- Write a C++ program that reads an upper bound and prints non-prime numbers within that range using a loop.
- Write a C++ program to list numbers between 1 and n that are not prime by checking for divisibility.
- Write a C++ program that outputs all non-prime numbers between 1 and a given limit and counts their total.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to asked user to input positive integers to process count, maximum, minimum,
and average or terminate the process with -1.
Next: Write a program in C++ to print a square pattern with # character.
What is the difficulty level of this exercise?