C++ Exercises: Print a square pattern with # character
C++ For Loop: Exercise-17 with Solution
Write a program in C++ to print a square pattern with the # character.
Visual Presentation:
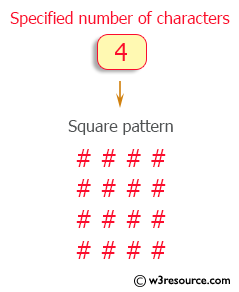
Sample Solution :-
C++ Code :
#include <iostream> // Include input-output stream header
using namespace std; // Using standard namespace to avoid writing std::
int main() // Start of the main function
{
int size; // Declaration of variable 'size' to store the number of characters for each side of the square
cout << "\n\n Print a pattern like square with # character:\n"; // Display message on the console
cout << "--------------------------------------------------\n";
cout << " Input the number of characters for a side: "; // Prompt the user to input the number of characters for a side
cin >> size; // Read the input value as the size of the square
// Loop for rows of the square
for (int row = 1; row <= size; ++row)
{
// Loop for columns of the square
for (int col = 1; col <= size; ++col)
{
cout << "# "; // Display the character '#' followed by a space to form the square pattern
}
cout << endl; // Move to the next line after printing a row of the square
}
return 0; // Indicates successful termination of the program
}
Sample Output:
Print a pattern like square with # character: -------------------------------------------------- Input the number of characters for a side: 4 # # # # # # # # # # # # # # # #
Flowchart:
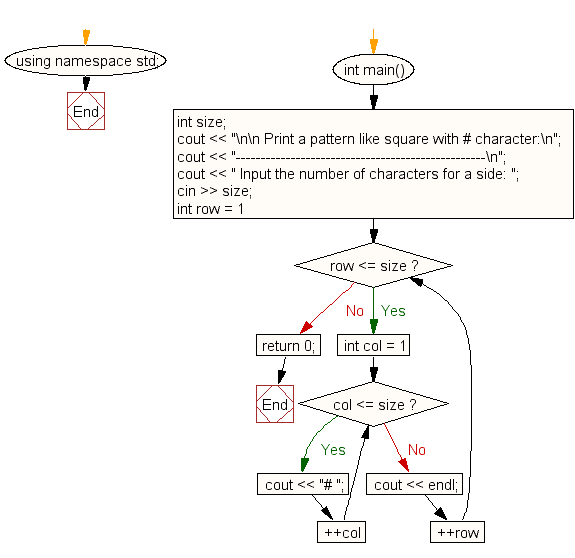
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to list non-prime numbers from 1 to an upperbound.
Next: Write a program in C++ to display the cube of the number upto given an integer.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/for-loop/cpp-for-loop-exercise-17.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics